How do you call a 2D array in C++?
Table of Contents
How do you call a 2D array in C++?
Passing two dimensional array to a C++ function
- Specify the size of columns of 2D array void processArr(int a[][10]) { // Do something }
- Pass array containing pointers void processArr(int *a[10]) { // Do Something } // When callingint *array[10]; for(int i = 0; i < 10; i++) array[i] = new int[10]; processArr(array);
How do you pass a 2D array to a function in CPP?
There are three ways to pass a 2D array to a function:
- The parameter is a 2D array int array[10][10]; void passFunc(int a[][10]) { // …
- The parameter is an array containing pointers int *array[10]; for(int i = 0; i < 10; i++) array[i] = new int[10]; void passFunc(int *a[10]) //Array containing pointers { // …
Can you pass arrays by reference C++?
Arrays can be passed by reference OR by degrading to a pointer.

How do you pass a 2D array to a function with pointers?
- #include
- // Here, the parameter is an array of pointers. void assign(int** arr, int m, int n)
- { for (int i = 0; i < m; i++)
- { for (int j = 0; j < n; j++) {
- arr[i][j] = i + j; }
- } }
- // Program to pass the 2D array to a function in C.
- int main(void) {
How do you input a 2D vector?
“how to take input from user in 2d vector in c++” Code Answer’s
- std::vector> d;
- //std::vector d;
- cout<<“Enter the N number of ship and port:”<
- cin>>in;
- cout<<“\Enter preference etc..:\n”;
- for(i=0; i
- cout<<“ship”<
- for(j=0; j
What is the use of Is_array () function in C++?
Explanation: is_array() function is used to check whether a given variable is of array type or not.
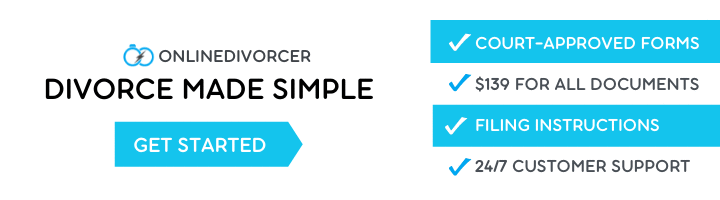
How do you pass a 2d vector into a function?
You can pass by value or by reference, or by pointer(not recommended). If you’re passing to a function that doesn’t change the contents, you can either pass by value, or by const reference.
Is array call by reference?
If we pass the address of an array while calling a function, then this is called function call by reference. The function declaration should have a pointer as a parameter to receive the passed address, when we pass an address as an argument.
How do you reference an array in C++?
Reference to an array will not be an int* but an int[]….
- The size needs to be mentioned as int[x] and int[y] are different data types from the compiler’s perspective.
- Reference to array needs to be initialized at the time of declaration.
- (&name) is not redundant. It has its own meaning.
How do you access the elements of a 2d array using pointer?
Pointers and two dimensional Arrays: In a two dimensional array, we can access each element by using two subscripts, where first subscript represents the row number and second subscript represents the column number. The elements of 2-D array can be accessed with the help of pointer notation also.
Is there any way of passing 2d array to a function without knowing any of its dimensions?
A short answer is “no”.
Can I return a vector in C++?
Yes, a function can return a vector in C++ and in different ways. This article explains the different ways in which a C++ function can return a vector. In order to code a vector in C++, the vector library has to be included in the program.
What is the use of Is_same () function in C++?
Explanation: is_same() function is used to check whether two variables have the same characteristics or not.
What is array manipulation in C++?
Array Type Manipulation in C++ The array is a data structure in c++ that stored multiple data elements of the same data type in continuous memory locations. In c++ programming language, there are inbuilt functions to manipulate array types. Some functions can also be applied to multidimensional arrays.
Can you pass a vector by reference?
A vector is not same as int[] (to the compiler). vector is non-array, non-reference, and non-pointer – it is being passed by value, and hence it will call copy-constructor. So, you must use vector& (preferably with const , if function isn’t modifying it) to pass it as a reference.
How do you pass an array of vector by reference?
Use the vector &arr Notation to Pass a Vector by Reference in C++ std::vector is a common way to store arrays in C++, as they provide a dynamic object with multiple built-in functions for manipulating the stored elements.
How does C++ pass by reference work?
How Does Pass By Reference Work With Functions? If you recall, using pass by reference allows us to effectively “pass” the reference of a variable in the calling function to whatever is in the function being called. The called function gets the ability to modify the value of the argument by passing in its reference.