How do you slice a multidimensional list in Python?
Table of Contents
How do you slice a multidimensional list in Python?
As shown in the above syntax, to slice a Python list, you have to append square brackets in front of the list name. Inside square brackets you have to specify the index of the item where you want to start slicing your list and the index + 1 for the item where you want to end slicing.
Can list allow multi dimensional in Python?
There can be more than one additional dimension to lists in Python. Keeping in mind that a list can hold other lists, that basic principle can be applied over and over. Multi-dimensional lists are the lists within lists.
Is slicing possible in list in Python?

With this operator, one can specify where to start the slicing, where to end, and specify the step. List slicing returns a new list from the existing list. If Lst is a list, then the above expression returns the portion of the list from index Initial to index End, at a step size IndexJump.
What is list slicing in Python with example?
It’s possible to “slice” a list in Python. This will return a specific segment of a given list. For example, the command myList[2] will return the 3rd item in your list (remember that Python begins counting with the number 0).
Is NumPy slicing inclusive?
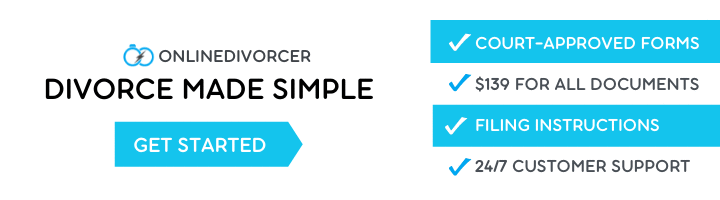
Slicing a One-dimensional Array Index ‘3’ represents the starting element of the slice and it’s inclusive.
How do you make a 3-dimensional list in Python?
How to create a 3D array in Python
- Use [] to create an empty list and assign it to the variable a_3d_list to hold the 3D list.
- Use a for-loop to iterate x times. In each iteration, use list.
- Inside this for-loop, use another for-loop to iterate y times.
- Inside the inner for-loop, use another for-loop to iterate z times.
How do I create a dynamic multidimensional list in Python?
“python create dynamic 2d array” Code Answer
- def build_matrix(rows, cols):
- matrix = []
- for r in range(0, rows):
- matrix. append([0 for c in range(0, cols)])
- return matrix.
- if __name__ == ‘__main__’:
Is list slicing fast in Python?
Python slicing is a computationally fast way to methodically access parts of your data. The colons (:) in subscript notation make slice notation – which has the optional arguments, start, stop, step.
Can you slice lists?
As well as using slicing to extract part of a list (i.e. a slice on the right hand sign of an equal sign), you can set the value of elements in a list by using a slice on the left hand side of an equal sign. In python terminology, this is because lists are mutable objects, while strings are immutable.
What are list slices explain with proper example?
List slicing refers to accessing a specific portion or a subset of the list for some operation while the orginal list remains unaffected. The slicing operator in python can take 3 parameters out of which 2 are optional depending on the requirement.
How do you slice a 2d array?
To slice elements from two-dimensional arrays, you need to specify both a row index and a column index as [row_index, column_index] . For example, you can use the index [1,2] to query the element at the second row, third column in precip_2002_2013 .
How do you create a multi dimensional array in Python?
In Python, Multidimensional Array can be implemented by fitting in a list function inside another list function, which is basically a nesting operation for the list function. Here, a list can have a number of values of any data type that are segregated by a delimiter like a comma.
How do you access a 3 dimensional array in Python?
The following code creates a 3-dimensional array:
- # a 3D array, shape-(2, 2, 2) >>> d3_array = np.
- # retrieving a single element from a 3D-array >>> d3_array[0, 1, 0] 2.
- # retrieving a 1D sub-array from a 3D-array >>> d3_array[:, 0, 0] array([0, 4])
What is the difference between slicing and indexing of list?
“Indexing” means referring to an element of an iterable by its position within the iterable. “Slicing” means getting a subset of elements from an iterable based on their indices.
Is slicing inclusive?
The slice() method selects from a given start, up to a (not inclusive) given end. The slice() method does not change the original array.
What is the purpose of slicing in Python?
Slicing in Python is a feature that enables accessing parts of sequences like strings, tuples, and lists. You can also use them to modify or delete the items of mutable sequences such as lists. Slices can also be applied on third-party objects like NumPy arrays, as well as Pandas series and data frames.
How do slices work in Python?
Python slice() Function The slice() function returns a slice object. A slice object is used to specify how to slice a sequence. You can specify where to start the slicing, and where to end. You can also specify the step, which allows you to e.g. slice only every other item.