Can I catch throwable in Java?
Table of Contents
Can I catch throwable in Java?
Don’t Catch Throwable Throwable is the superclass of all exceptions and errors. You can use it in a catch clause, but you should never do it! If you use Throwable in a catch clause, it will not only catch all exceptions; it will also catch all errors.
Should I catch exception or throwable?
Throwable is super class of Exception as well as Error . In normal cases we should always catch sub-classes of Exception , so that the root cause doesn’t get lost. Only special cases where you see possibility of things going wrong which is not in control of your Java code, you should catch Error or Throwable .
How do you use throwable in Java?

The Throwable class is the superclass of all errors and exceptions in the Java language. Only objects that are instances of this class (or one of its subclasses) are thrown by the Java Virtual Machine or can be thrown by the Java throw statement.
Can we use try catch and throws together?
Q #2) Can we use throws, try and catch in a single method? Answer: No. You cannot throw the exception and also catch it in the same method. The exception that is declared using throws is to be handled in the calling method that calls the method that has thrown the exception.
How do you catch and throw an exception?
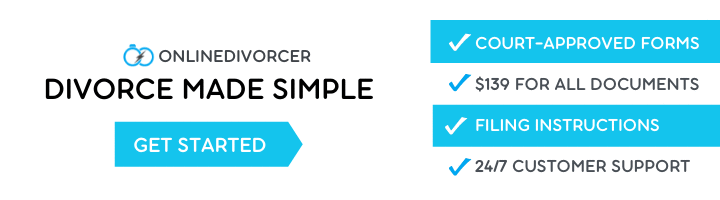
A thrown object may match several catch block but only the first catch block that matches the object will be executed. A catch-block will catch a thrown exception if and only if: the thrown exception object is the same as the exception object specified by the catch-block.
Can we use throws throwable?
You should not throw Throwable . Here’s why. Throwable is the top of the hierarchy of things that can be thrown and is made up of Exceptions and Errors . Since Errors by definition arise from unsalvagable conditions, it is pointless to include them in your method declaration.
What are try and catch in Java?
Java try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block.
What is the difference between try catch and throws Java?
Try-catch block is used to handle the exception. In a try block, we write the code which may throw an exception and in catch block we write code to handle that exception. Throw keyword is used to explicitly throw an exception. Generally, throw keyword is used to throw user defined exceptions.
Can we use throws for runtime exception?
Generally speaking, do not throw a RuntimeException or create a subclass of RuntimeException simply because you don’t want to be bothered with specifying the exceptions your methods can throw.
What is the difference between try-catch and throws Java?
How do you catch exception in Java?
The try-catch is the simplest method of handling exceptions. Put the code you want to run in the try block, and any Java exceptions that the code throws are caught by one or more catch blocks. This method will catch any type of Java exceptions that get thrown. This is the simplest mechanism for handling exceptions.
What is the difference between try catch and throws?
What is throw and throws in Java?
Throw is a keyword which is used to throw an exception explicitly in the program inside a function or inside a block of code. Throws is a keyword used in the method signature used to declare an exception which might get thrown by the function while executing the code.
What is try catch in Java?
What is the difference between throw throws and throwable in Java?
The throw and throws is the concept of exception handling where the throw keyword throw the exception explicitly from a method or a block of code whereas the throws keyword is used in signature of the method.
Can we use both throws and try catch?
From what I’ve read myself, the throws should be used when the caller has broken their end of the contract (passed object) and the try-catch should be used when an exception takes place during an operation that is being carried out inside the method.
Why try catch is used in Java?