How do I create a list in Scala?
Table of Contents
How do I create a list in Scala?
Syntax for defining a Scala List. val variable_name: List[type] = List(item1, item2, item3) or val variable_name = List(item1, item2, item3) A list in Scala is mostly like a Scala array. However, the Scala List is immutable and represents a linked list data structure. On the other hand, Scala array is flat and mutable.
How do I find the length of a list in Scala?
length function in Scala returns the number of elements in a list. In short, this function is used to get the current size of a list.
How do I fill a list in Scala?

Uniform List can be created in Scala using List. fill() method. List. fill() method creates a list and fills it with zero or more copies of an element.
How do you create an empty list in Scala?
Scala lists are immutable by default. You cannot “add” an element, but you can form a new list by appending the new element in front. Since it is a new list, you need to reassign the reference (so you can’t use a val).
How do I create a mutable list in Scala?
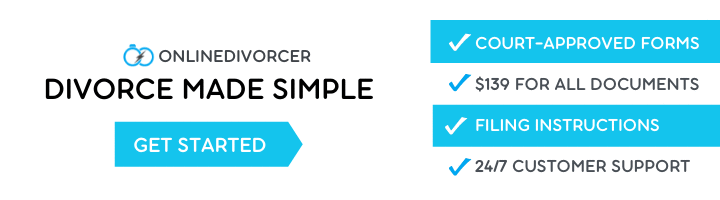
Because a List is immutable, if you need to create a list that is constantly changing, the preferred approach is to use a ListBuffer while the list is being modified, then convert it to a List when a List is needed. The ListBuffer Scaladoc states that a ListBuffer is “a Buffer implementation backed by a list.
How do you create an array in Scala?
In Scala arrays are immutable objects. You create an array like this: var myArray : Array[String] = new Array[String](10); First you declare variable var myArray to be of type Array[String] .
How do I find the length of a string in Scala?
Get length of the string So, a length() method is the accessor method in Scala, which is used to find the length of the given string. Or in other words, length() method returns the number of characters that are present in the string object. Syntax: var len1 = str1.
How do I fill an array in Scala?
Fill an Array in Scala
- Creating Array in Scala.
- Creating and Filling Array Using the fill Keyword in Scala.
- Creating and Filling Array Random Values in Scala.
- Creating and Filling Array Using the list() Elements in Scala.
- Creating and Filling Array Using the ofDim Keyword in Scala.
What is the difference between SEQ and list in Scala?
A Seq is an Iterable that has a defined order of elements. Sequences provide a method apply() for indexing, ranging from 0 up to the length of the sequence. Seq has many subclasses including Queue, Range, List, Stack, and LinkedList. A List is a Seq that is implemented as an immutable linked list.
What is difference between list and ListBuffer in Scala?
List is a functional data structure (immutability) compared to LinkedList which is more standard in imperative languages. ListBuffer – A mutable buffer implementation backed by a List . They both offer similar complexity bounds on most operations.
What is mutable list in Scala?
A MutableList consists of a single linked list together with a pointer that refers to the terminal empty node of that list. This makes list append a constant time operation because it avoids having to traverse the list in search for its terminal node. MutableList is currently the standard implementation of mutable.
What is the difference between SEQ and list in scala?
How do you use a range in scala?
The Range in Scala can be defined as an organized series of uniformly separated Integers….Operations performed on Ranges
- If we want a range inclusive of the end value, we can also use the until method both until and Range methods are used for the same purpose.
- The upper bound of the Range can be made inclusive.
How do I count characters in Scala?
The count() method in Scala is used to count the occurrence of characters in the string. The function will return the count of a specific character in the string.
How do I find a character in a string in Scala?
Scala String indexOf() method with example The indexOf() method is utilized to find the index of the first appearance of the character in the string and the character is present in the method as argument. Return Type: It returns the index of the character stated in the argument.
What is ::: in Scala?
The ::: operator in Scala is used to concatenate two or more lists. Then it returns the concatenated list.
How do you create a range array?
Steps to Add a Range into an Array in VBA
- First, you need to declare a dynamic array using the variant data type.
- Next, you need to declare one more variable to store the count of the cells from the range and use that counter for the loop as well.
- After that, assign the range where you have value to the array.
Is Scala list a seq?
In Java terms, Scala’s Seq would be Java’s List , and Scala’s List would be Java’s LinkedList . Note that Seq is a trait , which is equivalent to Java’s interface , but with the equivalent of up-and-coming defender methods.