How do you catch ExecutionException?
Table of Contents
How do you catch ExecutionException?
You can catch ExecutionException and just throw e. getCause — and don’t catch anything else, just let it propagate on its own.
What is Java Util Concurrent TimeoutException?
java.util.concurrent.TimeoutException. Exception thrown when a blocking operation times out. Blocking operations for which a timeout is specified need a means to indicate that the timeout has occurred.
What is the use of InterruptedException in Java?
Class InterruptedException. Thrown when a thread is waiting, sleeping, or otherwise occupied, and the thread is interrupted, either before or during the activity. Occasionally a method may wish to test whether the current thread has been interrupted, and if so, to immediately throw this exception.

Is runtime exception a subclass of exception?
RuntimeException is the superclass of those exceptions that can be thrown during the normal operation of the Java Virtual Machine. RuntimeException and its subclasses are unchecked exceptions.
Is TimeoutException an IOException?
You use a TimeoutException ; it most accurately conveys what actually happened. The IOException is more of a general-purpose exception; if something happened while reading the underlying backing store for the Stream for which there’s not a specific exception then I’d expect an IOException .
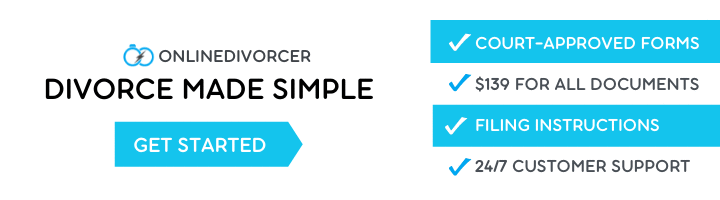
What is Java Util Concurrent ExecutionException?
java.util.concurrent.ExecutionException. Exception thrown when attempting to retrieve the result of a task that aborted by throwing an exception. This exception can be inspected using the Throwable.
What is the difference between IOException and exception?
Error , Exception , and RuntimeException all have several subclasses. For example, IOException is a subclass of Exception and NullPointerException is a subclass of RuntimeException . You may have noticed that Java differentiates errors from exceptions.
What is callable interface?
Interface Callable Implementors define a single method with no arguments called call. The Callable interface is similar to Runnable , in that both are designed for classes whose instances are potentially executed by another thread. A Runnable, however, does not return a result and cannot throw a checked exception.
How do I generate InterruptedException?
2 Answers
- Set up a new thread which will sleep for a short time, then interrupt the main thread.
- Start that new thread.
- Sleep for a long-ish time (in the main thread)
- Print out a diagnostic method when we’re interrupted (again, in the main thread)
How do I fix NullPointerException?
In Java, the java. lang. NullPointerException is thrown when a reference variable is accessed (or de-referenced) and is not pointing to any object. This error can be resolved by using a try-catch block or an if-else condition to check if a reference variable is null before dereferencing it.
What is the purpose of IOException?
IOException is usually a case in which the user inputs improper data into the program. This could be data types that the program can’t handle or the name of a file that doesn’t exist. When this happens, an exception (IOException) occurs telling the compiler that invalid input or invalid output has occurred.
What is IOException in Java?
IOException. This exception is related to Input and Output operations in the Java code. It happens when there is a failure during reading, writing, and searching file or directory operations. IOException is a checked exception. A checked exception is handled in the java code by the developer.
What is phaser in Java?
public class Phaser extends Object. A reusable synchronization barrier, similar in functionality to CyclicBarrier and CountDownLatch but supporting more flexible usage. Registration. Unlike the case for other barriers, the number of parties registered to synchronize on a phaser may vary over time.