How do you call a CSS class in JavaScript?
Table of Contents
How do you call a CSS class in JavaScript?
className = “MyClass”; In the css class MyClass you can set the background image.. You can create another css class with different background image and set it according to the conditions.. Show activity on this post.
How can you add a class name to addBtn?
“How can you add a class name to addBtn in javascript” Code Answer’s
- var element = document. getElementById(‘element’);
- element. classList. add(‘class-1’);
- element. classList. add(‘class-2’, ‘class-3’);
- element. classList. remove(‘class-3’);
How do you add a class?

2 different ways to add class using JavaScript
- Using element.classList.add() Method. var element = document. querySelector(‘.box’); // using add method // adding single class element.
- Using element. className Property. Note: Always use += operator and add a space before class name to add class with classList method.
Can I add a class to an element using CSS?
With CSS Hero you can easily add classes to elements without needing to touch any of your theme files or adding weird code to your contents. Plus you can control all your classes right from the CSS Hero interface.
How do you reference a CSS class in HTML?
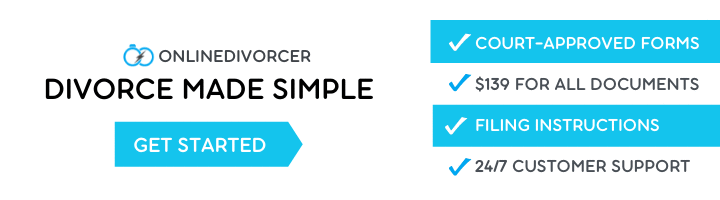
To select elements with a specific class, write a period (.) character, followed by the name of the class. You can also specify that only specific HTML elements should be affected by a class. To do this, start with the element name, then write the period (.)
How can you add a class name to button?
Add class to clicked Element using JavaScript #
- Add a click event listener on the document object.
- Use the target property on the event object to get the clicked element.
- Use the classList. add() method to add a class to the element.
How do I add a class in Dom?
Following are the properties of Javascript that we will be using to add a class to the DOM element:
- classList Property: It returns the class name as a DOMTokenList object. It has a method called “add” which is used to add class name to elements.
- className Property: This property returns the className of the element.
How do you add a name to a class?
“append class name javascript” Code Answer’s
- var element = document. getElementById(‘element’);
- element. classList. add(‘class-1’);
- element. classList. add(‘class-2’, ‘class-3’);
- element. classList. remove(‘class-3’);
How do you call a CSS class on button click?
“add css class on button click javascript” Code Answer
- var element = document. getElementById(‘element’);
- element. classList. add(‘class-1’);
- element. classList. add(‘class-2’, ‘class-3’);
- element. classList. remove(‘class-3’);
How can you add a class name to a button?
How do you assign a class in JavaScript?
1. Add class
- Method 1: Best way to add class in the modern browser is using classList. add() method of element.
- Method 2 – You can also add classes to HTML elements using className property.
- Method 1 – Best way to remove a class from an element is classList.
- Method 2 – You can also remove class using className method.
How can CSS classes be manipulated in HTML using jQuery?
The jQuery CSS methods allow you to manipulate CSS class or style properties of DOM elements. Use the selector to get the reference of an element(s) and then call jQuery css methods to edit it. Important DOM manipulation methods: css(), addClass(), hasClass(), removeClass(), toggleClass() etc.