How to append data to StringBuilder in c#?
Table of Contents
How to append data to StringBuilder in c#?
Use the Append() method to add or append strings to the StringBuilder object. Use the ToString() method to retrieve a string from the StringBuilder object.
How to append value in c#?
Here are the 6 ways to concatenate strings in C#.
- Using + operator.
- String Interpolation.
- String.Concatenate() method.
- String.Join() method.
- String.Format() method.
- StringBuilder.Append() method.
How to add in string Builder?
Example of Java StringBuilder append(String str) method

- public class StringBuilderAppendExample12 {
- public static void main(String[] args) {
- StringBuilder sb = new StringBuilder(“append string “);
- System.out.println(“builder :”+sb);
- String str =”my string”;
- // appending string argument.
- sb.append(str);
What is difference between append and AppendLine in StringBuilder C#?
Append: Appends information to the end of the current StringBuilder. AppendLine: Appends information as well as the default line terminator to the end of the current StringBuilder.
What is StringBuilder C#?
The C# StringBuilder class of . NET is a class to work with strings when repetitive string operations are needed. Use of strings in . NET could be a costly affair if not used property. Strings being immutable objects, any modification of a string object creates a new string object.
Why StringBuilder is faster than string?
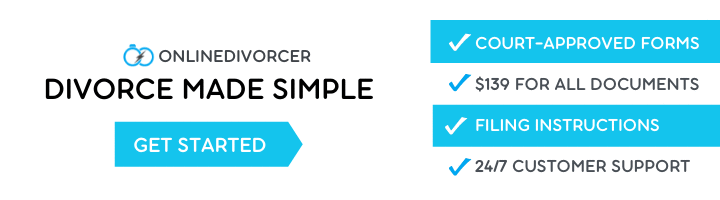
String is immutable whereas StringBuffer and StringBuilder are mutable classes. StringBuffer is thread-safe and synchronized whereas StringBuilder is not. That’s why StringBuilder is faster than StringBuffer.
How many times is the StringBuilder append method overloaded?
Append() in StringBuilder and StringBuffer There are 13 various overloaded append() methods in both StringBuffer and StringBuilder classes.
Is StringBuilder mutable in C#?
StringBuilder is a mutable string in C#. With StringBuilder, you can expand the number of characters in the string. The string cannot be changed once it is created, but StringBuilder can be expanded. It does not create a new object in the memory.
Why do we use StringBuilder in C#?
StringBuilder class can be used when you want to modify a string without creating a new object. For example, using the StringBuilder class can boost performance when concatenating many strings together in a loop.
Does StringBuilder use array?
The StringBuilder works by maintaining a buffer of characters (Char) that will form the final string. Characters can be appended, removed and manipulated via the StringBuilder, with the modifications being reflected by updating the character buffer accordingly. An array is used for this character buffer.
Is StringBuilder better than concatenation?
It is always better to use StringBuilder. append to concatenate two string values.
Which is faster string or StringBuilder C#?
StringBuilder has overhead, so string is faster for limited concatenations. If you are going to append or modify thousands of times (usually not the case) then StringBuilder is faster in those scenarios.
Which is better string or StringBuilder C#?
StringBuilder is used to represent a mutable string of characters. Mutable means the string which can be changed. So String objects are immutable but StringBuilder is the mutable string type. It will not create a new modified instance of the current string object but do the modifications in the existing string object.