How do you find the inorder traversal of a binary tree?
Table of Contents
How do you find the inorder traversal of a binary tree?
Inorder Traversal Iterative
- Create a stack to store the values while traversing to the leftmost child.
- Create a current node as root.
- Traverse till current node is not null or we have elements in our stack to process.
- As in order, we need the leftmost child first, so traverse to get the leftmost child in a nested loop.
What is inorder traversal of a binary tree?
3. Inorder Traversal. An inorder traversal first visits the left child (including its entire subtree), then visits the node, and finally visits the right child (including its entire subtree). The binary search tree makes use of this traversal to print all nodes in ascending order of value.
How can you traverse binary tree?
In-order Traversal In this traversal method, the left subtree is visited first, then the root and later the right sub-tree. We should always remember that every node may represent a subtree itself. If a binary tree is traversed in-order, the output will produce sorted key values in an ascending order.

What is the inorder traversal of this tree example?
Example of inorder traversal The nodes with yellow color are not visited yet. Now, we will traverse the nodes of the above tree using inorder traversal. Here, 40 is the root node. We move to the left subtree of 40, that is 30, and it also has subtree 25, so we again move to the left subtree of 25 that is 15.
Is inorder traversal sorted?
The inOrder traversal literally means IN order, i.e notes are printed in the order or sorted order. Even though these three algorithms (pre-order, in-order, and post-order) are popular binary tree traversal algorithms, they are not the only ones.
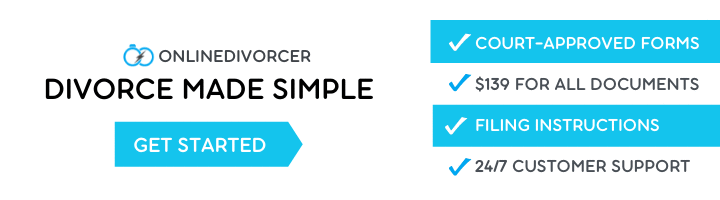
What is the use of inOrder traversal?
In-order traversal is very commonly used in binary search trees because it returns values from the underlying set in order, according to the comparator that set up the binary search tree (hence the name). Post-order traversal while deleting or freeing nodes and values can delete or free an entire binary tree.
What is the inOrder traversal of this tree example?
Example of inorder traversal we start recursive call from 30(root) then move to 20 (20 also have sub tree so apply in order on it),15 and 5. 5 have no child . so print 5 then move to it’s parent node which is 15 print and then move to 15’s right node which is 18. 18 have no child print 18 and move to 20 .
Is inOrder traversal of binary search tree sorted?
It’s worth remembering that the inOrder traversal is a depth-first algorithm and prints the tree node in sorted order if given binary tree is a binary search tree.
What is true about inorder traversal of tree?
In the case of binary search trees (BST), Inorder traversal gives nodes in non-decreasing order. To get nodes of BST in non-increasing order, a variation of Inorder traversal where Inorder traversal s reversed can be used.
What are types of binary tree traversal?
4 Types of Tree Traversal Algorithms. Everything you need to know about tree traversal in 7 mins (with animations)
Is inorder traversal always sorted?
The Inorder traversal of a BST is always in sorted or increasing order.
What is the use of inorder traversal?