Which is faster Enumeration or Iterator?
Table of Contents
Which is faster Enumeration or Iterator?
Iterator is used to iterate most of the classes in the collection framework like ArrayList, HashSet, HashMap, LinkedList etc. Enumeration is fail-safe in nature. Iterator is fail-fast in nature. Enumeration is not safe and secured due to it’s fail-safe nature.
What is the difference between Iterator and Enumeration in Java?
In Iterator, we can read and remove element while traversing element in the collections. Using Enumeration, we can only read element during traversing element in the collections. 2. It can be used with any class of the collection framework.
Which is the fastest loop in Java?
Iterator and for-each loop are faster than simple for loop for collections with no random access, while in collections which allows random access there is no performance change with for-each loop/for loop/iterator.

Is Enumeration fail-fast?
Fail-fast or Fail-safe : Enumeration is fail-safe in nature. It does not throw ConcurrentModificationException if Collection is modified during the traversal. Iterator is fail-fast in nature. It throws ConcurrentModificationException if a Collection is modified while iterating other than its own remove() method.
Which Iterator is faster and uses less memory in Java?
Enumeration
Enumeration is twice as fast as Iterator and uses very little memory.
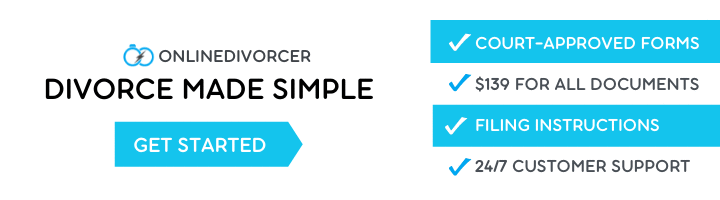
What is difference between Iterator and enumerator?
Iterator can do modifications (e.g using remove() method it removes the element from the Collection during traversal). Enumeration interface acts as a read only interface, one can not do any modifications to Collection while traversing the elements of the Collection.
Is iterator slow Java?
Iterator is faster for collections with no random access (e.g. TreeSet, HashMap, LinkedList). For arrays and ArrayLists, performance differences should be negligible.
How can loop performance be improved?
The best ways to improve loop performance are to decrease the amount of work done per iteration and decrease the number of loop iterations. Generally speaking, switch is always faster than if-else , but isn’t always the best solution.
Which collections are fail-fast in Java?
Default iterators for Collections from java. util package such as ArrayList, HashMap, etc. are Fail-Fast. In the code snippet above, the ConcurrentModificationException gets thrown at the beginning of a next iteration cycle after the modification was performed.
Which is faster and uses less memory Iterator enumeration?
Enumeration is twice as fast as Iterator and uses very little memory.
Why Iterator is fail-fast?
As name suggest fail-fast Iterators fail as soon as they realized that structure of Collection has been changed since iteration has begun. Structural changes means adding, removing or updating any element from collection while one thread is Iterating over that collection.
Which feature is most important Java?
The most significant feature of Java is that it provides platform independence which leads to a facility of portability, which ultimately becomes its biggest strength. Being platform-independent means a program compiled on one machine can be executed on any machine in the world without any change.
Is enumerate an Iterator?
In this example, you assign the return value of enumerate() to enum . enumerate() is an iterator, so attempting to access its values by index raises a TypeError . Fortunately, Python’s enumerate() lets you avoid all these problems.
Why are iterators faster?
Iterators is a generic concept. They work on all sorts of containers and have similar interface. Accessing the array element directly like arr_int[i] is definitely faster because it directly translates to pointer arithmetic.
Which loop is best in Java?
Java for Loop vs while Loop vs do-while Loop
Comparison | for loop |
---|---|
Introduction | The Java for loop is a control flow statement that iterates a part of the programs multiple times. |
When to use | If the number of iteration is fixed, it is recommended to use for loop. |
Syntax | for(init;condition;incr/decr){ // code to be executed } |
What is faster than for loop in Java?
get(i); runs faster than this loop: for (Iterator i=list.
Is ConcurrentHashMap fail-safe?
concurrent package such as ConcurrentHashMap, CopyOnWriteArrayList, etc. are Fail-Safe in nature. In the code snippet above, we’re using Fail-Safe Iterator. Hence, even though a new element is added to the Collection during the iteration, it doesn’t throw an exception.