How do you make a file into a string in Python?
Table of Contents
How do you make a file into a string in Python?
Generally, to read file content as a string, follow these steps.
- Open file in read mode. Call inbuilt open() function with file path as argument.
- Call read() method on the file object. read() method returns whole content of the file as a string.
- Close the file by calling close() method on the file object.
How do I read a text file into a string?
The file object provides you with three methods for reading text from a text file:
- read() – read all text from a file into a string.
- readline() – read the text file line by line and return all the lines as strings.
- readlines() – read all the lines of the text file and return them as a list of strings.
How do I put contents of a text file into a variable in Python?
The file read() method can be used to read the whole text file and return as a single string. The read text can be stored into a variable which will be a string. Alternatively the file content can be read into the string variable by using the with statement which do not requires to close file explicitly.

How do I turn a file into a variable in Python?
Here are the steps to read file into string in python.
- Open the file in read mode using open() method and store it in variable named file.
- Call read() function on file variable and store it into string variable countriesStr .
- print countriesStr variable.
How do I read a file path to a string in Python?
Use the pathlib. read_text() Function to Read a Text File to a String in Python. The pathlib module is added to Python 3.4 and has more efficient methods available for file-handling and system paths. The read_text() function from this module can read a text file and close it in the same line.
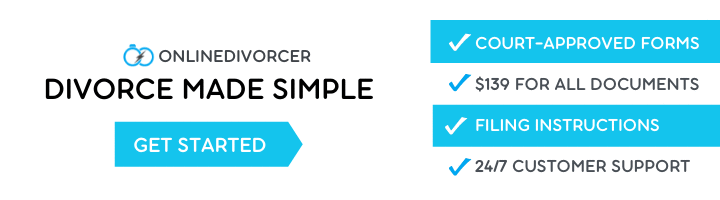
How do you make a file into a list in Python?
In Python 3.4. , we can use the Pathlib module to convert a file to a list. This is a simple way to convert a file to a list. In this example, we will use the read_text() method to read the file and the splitlines() method for the splitting of lines.
How do you convert an int to a string in Python?
To convert an integer to string in Python, use the str() function. This function takes any data type and converts it into a string, including integers. Use the syntax print(str(INT)) to return the int as a str , or string.
How do you open a file and search for a string in Python?
Steps:
- Open a file.
- Set variables index and flag to zero.
- Run a loop through the file line by line.
- In that loop check condition using the ‘in’ operator for string present in line or not. If found flag to 0.
- After loop again check condition for the flag is set or not.
- Close a file.
How do you create a dataset from a text file?
To create a dataset using a local text file data source, identify the location of the file, and then upload it. The file data is automatically imported into SPICE as part of creating a dataset. Check Data source quotas to make sure that your target file doesn’t exceed data source quotas. Supported file types include .
How do you convert a file into a list?
9 ways to convert file to list in Python
- Pathlib to Convert text file to list Python.
- For Loop to split file to list Python.
- File.
- Readline() to create list from text file.
- Using iter() to read and storetext file in list Python.
- Python read text file line by line into list Python.
How do I turn a text file into a list?
Use str. split() to convert each line in a text file into a list
- a_file = open(“sample.txt”, “r”)
- list_of_lists = []
- for line in a_file:
- stripped_line = line. strip()
- line_list = stripped_line. split()
- list_of_lists. append(line_list)
- a_file. close()
- print(list_of_lists)