Does C++ have a max-heap?
Table of Contents
Does C++ have a max-heap?
A Binary Heap is a complete binary tree which is either Min Heap or Max Heap. In a Max Binary Heap, the key at root must be maximum among all keys present in Binary Heap. This property must be recursively true for all nodes in Binary Tree.
Is C++ priority queue max-heap?
The top of the queue is the maximum element in the queue since priority_queue is implemented as max-heap by default.
How do I check my max-heap?
Program to check heap is forming max heap or not in Python

- n := size of nums.
- for i in range 0 to n – 1, do. m := i * 2. num := nums[i] if m + 1 < n, then. if num < nums[m + 1], then. return False. if m + 2 < n, then. if num < nums[m + 2], then. return False.
- return True.
How do you define max-heap in C++ STL?
A max-heap is a binary tree such that: 1. The data contained in each node is greater than (or equal to) the data in that node’s children. The C++ Standard Library consists of a container named priority_queue.
What is stack memory in C++?
In C++ the stack memory is where local variables get stored/constructed. The stack is also used to hold parameters passed to functions. The stack is very much like the std::stack class: you push parameters onto it and then call a function.
What is min-heap in C++?
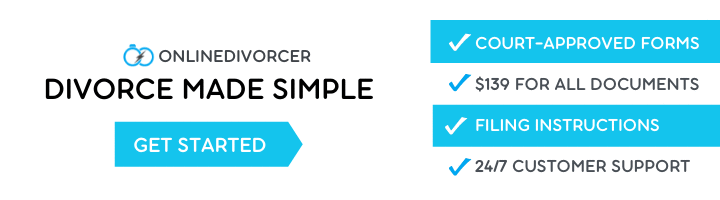
Minimum Heap is a method of arranging elements in a binary search tree where value of the parent node is lesser than that of it’s child nodes. Here is the source code of the C++ program to display the min heap after giving inputs of elements in array.
What is max-heap property?
the max-heap property: the value of each node is less than or equal to the value of its parent, with the maximum-value element at the root.
What is heap memory in C++?
Memory in your C++ program is divided into two parts − The stack − All variables declared inside the function will take up memory from the stack. The heap − This is unused memory of the program and can be used to allocate the memory dynamically when program runs.
Is there a heap in STL C++?
Heap data structure can be implemented in a range using STL which allows faster input into heap and retrieval of a number always results in the largest number i.e. largest number of the remaining numbers is popped out each time. Other numbers of the heap are arranged depending upon the implementation.
How large is the stack in C++?
1MB
The stack has a limited size, and consequently can only hold a limited amount of information. On Windows, the default stack size is 1MB. On some unix machines, it can be as large as 8MB. If the program tries to put too much information on the stack, stack overflow will result.
Does C++ have a heap?
Memory in a C/C++/Java program can either be allocated on a stack or a heap.
What is heap memory C++?
What do you mean by Max Heap?
A max-heap is a complete binary tree in which the value in each internal node is greater than or equal to the values in the children of that node. Mapping the elements of a heap into an array is trivial: if a node is stored an index k, then its left child is stored at index 2k + 1 and its right child at index 2k + 2.
What is a heap in C++?
A heap is a way to organize the elements of a range that allows for fast retrieval of the element with the highest value at any moment (with pop_heap), even repeatedly, while allowing for fast insertion of new elements (with push_heap). The element with the highest value is always pointed by first .