How do you traverse a given binary tree in preorder without recursion in Java?
Table of Contents
How do you traverse a given binary tree in preorder without recursion in Java?
Pre-order traversal in Java without recursion
- Create an empty stack.
- Push the root into Stack.
- Loop until Stack is empty.
- Pop the last node and print its value.
- Push right and left node if they are not null.
- Repeat from steps 4 to 6 again.
What is recursive and non recursive tree traversing?
Recursive functions are simpler to implement since you only have to care about a node, they use the stack to store the state for each call. Non-recursive functions have a lot less stack usage but require you to store a list of all nodes for each level and can be far more complex than recursive functions.
What is recursive and non-recursive tree traversing?
What is the difference between recursive and non-recursive function?

Recursive function is a function which calls itself again and again. A recursive function in general has an extremely high time complexity while a non-recursive one does not.
What is the difference between recursive and non recursive function?
How do you preorder traversal in data structure?
Algorithm for Preorder Traversal: for all nodes of the tree: Step 1: Visit the root node. Step 2: Traverse left subtree recursively. Step 3: Traverse right subtree recursively.
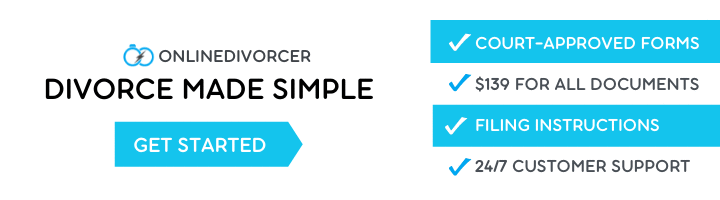
How do you pre-order traversal in a tree?
Now, let’s see the algorithm of preorder traversal.
- Step 1: Repeat Steps 2 to 4 while TREE != NULL.
- Step 2: Write TREE -> DATA.
- Step 3: PREORDER(TREE -> LEFT)
- Step 4: PREORDER(TREE -> RIGHT)
- [END OF LOOP]
- Step 5: END.
What is recursion and without recursion?
Recursive functions are procedures or subroutines implemented in a programming language, whose implementation references itself. Non Recursive Function are procedures or subroutines implemented in a programming language, whose implementation does not references itself.
Can you implement a binary search algorithm without recursion?
Yes, you guessed it right: you need to implement a binary search in Java, and you need to write both iterative and recursive binary search algorithms. In computer science, a binary search, or half-interval search, is a divide and conquer algorithm that locates the position of an item in a sorted array.