How do I encrypt a password in Windows PowerShell?
Table of Contents
How do I encrypt a password in Windows PowerShell?
Securely store passwords on a Windows disk
- In Windows PowerShell, use the ConvertFrom-SecureString cmdlet to convert a secure string into an encrypted plaintext string that can be written to disk and used later: $pw | ConvertFrom-SecureString.
- You can pipe this output directly into a file and know it is encrypted.
How do I securely use credentials in PowerShell?
Securely Storing Credentials with PowerShell
- Use Integrated Windows Authentication.
- Request the username and password every time you run the script.
- Store the username and password in the script itself.
- Store the username and password in a file protected with an ACL.
- Store the username and password in an encrypted file.
How do I encrypt and decrypt in PowerShell?
PowerShell Commands Used ConvertTo-SecureString – Decrypt data. Read-Host – Read input as a secure string. RNGCryptoServiceProvider. GetBytes – RNGCryptoServiceProvider’s GetBytes method will be used to create an encryption key which will be used for encryption and decryption.

How do I encrypt a PowerShell script?
Part One: Encrypt the Original Script In this block of code, we create a variable called $Code that contains the unencrypted contents of the text file. Next, we convert the script file into a secure string and store its contents in a variable called $CodeSecureString.
How do I create a Windows password encryption?
To use the Generate Encrypted Password window
- Type your password into the Password textbox.
- AQT will encrypt the password and show the result to you in Encrypted Password.
- AQT will then also decrypt the encrypted password and show this in Decrypt Check.
What encryption does PowerShell use?
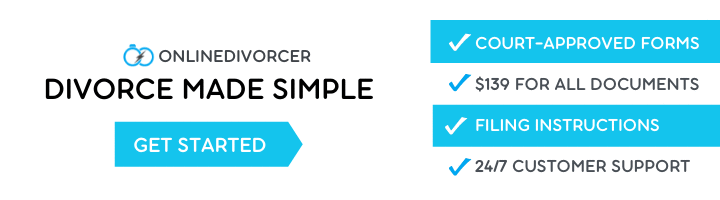
When you are not using the –Key or –SecureKey parameters, PowerShell uses the Windows Data Protection API to encrypt/decrypt your strings. This effectively means that only the same user account on the same computer will be able to use this encrypted string.
Are PowerShell commands encrypted?
PowerShell doesn’t have a built-in way to store encrypted text to the file system but it can encrypt text in memory. It’s just up to you to store it somewhere either in a file, a database, wherever. Encrypting strings in PowerShell comes in the form of the ConvertTo-SecureString cmdlet.
How do I create a credential file in PowerShell?
How to: How To – Create a Secure Credential file for use with PowerShell
- Step 1: On the host server, open PowerShell with Administrator access.
- Step 2: Type in the following command: read-host -assecurestring | convertfrom-securestring | out-file D:\Scripts\secure.txt.
How to secure your passwords with PowerShell?
First,save the PowerShell script below in a PS1 file (e.g. C:\\CreateSecurePassword.ps1).
How to show an encrypted password?
You try to brute force it. It could work.
How to display securestring password in PowerShell?
Prompting for credentials. Using Get-Credential in parentheses () at run time causes the Get-credential to run first.
How to use AES encryption in PowerShell?
Derive key from password. We can leverage the class Rfc2898DeriveBytes in .Net for this.