Is Tkinter multithreaded?
Table of Contents
Is Tkinter multithreaded?
With Tkinter, we can call multiple functions at a time using Threading. It provides asynchronous execution of some functions in an application. In order to use a thread in Python, we can import a module called threading and subclass its Thread class.
Can you run Tkinter in a thread?
Tkinter isn’t thread safe, and the general consensus is that Tkinter doesn’t work in a non-main thread. If you rewrite your code so that Tkinter runs in the main thread, you can have your workers run in other threads. The main caveat is that the workers cannot interact with the Tkinter widgets.
Is Tkinter single thread?

When to use Thread in Tkinter applications. In a Tkinter application, the main loop should always start in the main thread. It’s responsible for handling events and updating the GUI. If you have a background operation that takes time, you should execute it in a separate thread.
What is Python daemon thread?
The threads which are always going to run in the background that provides supports to main or non-daemon threads, those background executing threads are considered as Daemon Threads. The Daemon Thread does not block the main thread from exiting and continues to run in the background.
How do I open one GUI from another GUI in Python?
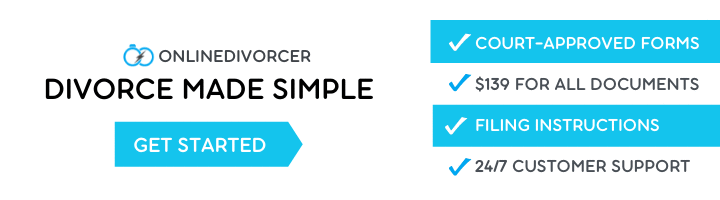
You can’t “call” another GUI. If this other GUI creates its own root window and calls mainloop() , your only reasonable option is to spawn a new process. That’s a simple solution that requires little work. The two GUIs will be completely independent of each other.
Why is Python not thread safe?
A lack of thread safety means that the methods/functions don’t have protection against multiple threads interacting with that data at the same time – they don’t have locks around data to ensure things are consistent. The async stuff isn’t thread safe because it doesn’t need to be.
What is single thread and multi thread in Python?
A thread is a lightweight process or task. A thread is one way to add concurrency to your programs. If your Python application is using multiple threads and you look at the processes running on your OS, you would only see a single entry for your script even though it is running multiple threads.
How do I open a second Tkinter window?
How to Open a New Window With a Button in Python Tkinter
- from tkinter import * def create():
- win = Toplevel(root) root = Tk()
- root. geometry(‘200×100’) btn = Button(root, text=”Create a new window”, command = create)
- btn. pack(pady = 10) root. mainloop()
What does pack () do in Tkinter?
The pack() fill option is used to make a widget fill the entire frame. The pack() expand option is used to expand the widget if the user expands the frame. fill options: NONE (default), which will keep the widget’s original size.
Is Python single-threaded or multithreaded?
Python is NOT a single-threaded language. Python processes typically use a single thread because of the GIL. Despite the GIL, libraries that perform computationally heavy tasks like numpy, scipy and pytorch utilise C-based implementations under the hood, allowing the use of multiple cores.
Is multithreading faster than multiprocessing?
For most problems, multithreading is probably significantly faster than using multiple processes, but as soon as you encounter hardware limitations, that answer goes out the window.
Is multiprocessing faster than multithreading in Python?
Another use case for threading is programs that are IO bound or network bound, such as web-scrapers. 2-Use Cases for Multiprocessing: Multiprocessing outshines threading in cases where the program is CPU intensive and doesn’t have to do any IO or user interaction.