How do I draw a line in OpenCV?
Table of Contents
How do I draw a line in OpenCV?
You can draw a line on an image using the method line() of the imgproc class. Following is the syntax of this method. mat − A Mat object representing the image on which the line is to be drawn.
How do you draw a line between points in OpenCV?
How to draw lines between points in OpenCV?…
- There’s a tutorial in the official documentation for drawing.
- I know, but tutorial tells to use two points: cv2.line(img,(0,0),(511,511),(255,0,0),5) , but I have some points.
- You can also draw polygons or contours using your point list.
- Please show some of your code.
How do you draw a line between two points in OpenCV Python?
line() method is used to draw a line on any image. Parameters: image: It is the image on which line is to be drawn. start_point: It is the starting coordinates of the line. The coordinates are represented as tuples of two values i.e. (X coordinate value, Y coordinate value).

How do you draw a line in Python?
Use matplotlib. pyplot. plot() to draw a line between two points
- point1 = [1, 2]
- point2 = [3, 4]
- x_values = [point1[0], point2[0]] gather x-values.
- y_values = [point1[1], point2[1]] gather y-values.
- plt. plot(x_values, y_values)
How do you draw a line in a video using OpenCV in Python?
Step 1: Import cv2. Step 2: Read the image using imread(). Step 3: Get the dimensions of the image using the image. shape method.
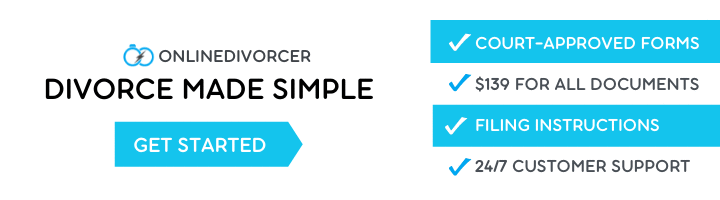
Which function is used to draw lines in OpenCV?
cv2.line() method
The cv2. line() method in the OpenCV is used to draw a line.
How do you draw a shape in OpenCV Python?
Some of the drawing functions are :
- line() : Used to draw line on an image.
- rectangle() : Used to draw rectangle on an image.
- circle() : Used to draw circle on an image.
- putText() : Used to write text on image.
How do you draw a rectangle in OpenCV Python?
Rectangle in OpenCV Python : cv2. rectangle()
- image – It is the image on which the rectangle has to be drawn.
- pt1 – Vertex of the rectangle at the top left corner.
- pt2 – Vertex of the rectangle at the bottom right corner.
- color – It is the color of the rectangle line in RGB.
- thickness – It is the thickness of the line.
How do you draw a horizontal line in Python?
In matplotlib, if you want to draw a horizontal line with full width simply use the axhline() method. You can also use the hlines() method to draw a full-width horizontal line but in this method, you have to set xmin and xmax to full width.
How do you draw a line in GUI in Python?
Programming code
- #Drawing basic line on the canvas.
- from tkinter import *
- from tkinter import ttk.
- app=Tk()
- #App Title.
- app.title(“Python GUI Application “)
- #Lable.
- name=ttk.Label(app, text=”Draw basic line”)
How do you draw OpenCV contours?
How to draw the contours?
- To draw all the contours in an image: cv.drawContours(img, contours, -1, (0,255,0), 3)
- To draw an individual contour, say 4th contour: cv.drawContours(img, contours, 3, (0,255,0), 3)
- But most of the time, below method will be useful: cnt = contours[4]
How do you draw a square in OpenCV in Python?
OpenCV allows a user to create a wide variety of shapes, including rectangles, squares, circles, etc. Python has a built-in rectangle() function, which allows us to add a square to an image, usually a blank one. We create this blank image with numpy. Then using OpenCV, we add our square shape.
How do you draw a rectangle shape in Python?
We can create a simple rectangle by defining a function that takes in two integers representing side length and side height. Then we can loop four times, using the forward() function to create a side representing either the length or height, then rotating the cursor 90 degrees with the right() function.
How do I make a line in Python?
In Python, the new line character “\n” is used to create a new line. When inserted in a string all the characters after the character are added to a new line. Essentially the occurrence of the “\n” indicates that the line ends here and the remaining characters would be displayed in a new line.
How do you draw a line in Python code?
How can I draw lines on a picture?
How to Use the Line Tool
- Upload an image using the form above to load it in the online editor. If you already have an image opened in the editor select the line tool by clicking its icon in the toolbar.
- To begin drawing press in the image to set the starting point of the line.
- Release the mouse to complete drawing.
How do you draw a line using PIL in Python?
line() Draws a line between the coordinates in the xy list. Parameters: xy – Sequence of either 2-tuples like [(x, y), (x, y), …] or numeric values like [x, y, x, y, …]. fill – Color to use for the line.
How do you draw a contour line in Python?
Density and Contour Plots
- %matplotlib inline import matplotlib.pyplot as plt plt. style.
- def f(x, y): return np. sin(x) ** 10 + np.
- x = np. linspace(0, 5, 50) y = np.
- plt. contour(X, Y, Z, colors=’black’);
- plt. contour(X, Y, Z, 20, cmap=’RdGy’);
- In [6]: plt.
- In [7]: plt.
- In [8]: contours = plt.