What is the stack size of a thread?
Table of Contents
What is the stack size of a thread?
The stack size is determined when the thread is created since it needs to occupy contiguous address space. That means that the entire address space for the thread’s stack has to be reserved at the point of creating the thread. If the stack is too small then it can overflow.
How many maximum threads can be created using a ThreadPool?
ThreadPool will create maximum of 10 threads to process 10 requests at a time. After process completion of any single Thread, ThreadPool will internally allocate the 11th request to this Thread and will keep on doing the same to all the remaining requests.
What is Java thread stack size?
The Java stack size is the size limit of each Java thread in the Java Virtual Machine (JVM) that runs the monitoring Model Repository Service. The default value is 512K. You can increase this property to make more memory available to monitoring Model Repository Service processes.
How do you calculate stack size?

Count the number of complete strings, multiply by 8 (since “STACK—” is 8 bytes long), and you have the number of bytes of remaining stack space.
What is thread stack?
A thread’s stack is used to store the location of function calls in order to allow return statements to return to the correct location. Since there usually is only one important call stack, it is what people refer to as the stack.
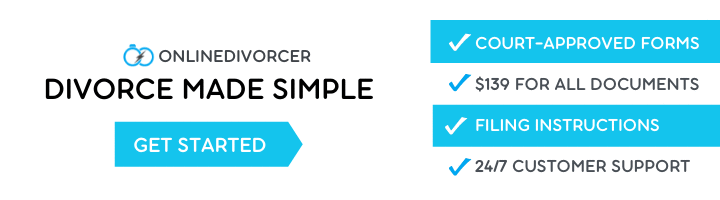
What is an ideal ThreadPool size?
Ideally, there is no fixed number which is ideal to be used in deciding the number of threads pool. It all depends on the use-case of the java program. Therefore, there are two factors on which decision should be taken to decide the thread pool size.
How do I know what size ThreadPool to get?
Just give me the formula!
- Number of threads = Number of Available Cores * (1 + Wait time / Service time)
- Number of threads = Number of Available Cores * Target CPU utilization * (1 + Wait time / Service time)
- 22 / 0.055 = 400 // the number of requests per second our service can handle with a stable response time.
What should be the return type of size in stack?
The number of elements in the stack is returned, which is a measure of the size of the stack. Hence the function has an integer return type as size is an int value.
What is the default capacity of stack?
Stack is 10. Default capacity of System.
How do you make a ThreadPool?
File: TestThreadPool.java
- public class TestThreadPool {
- public static void main(String[] args) {
- ExecutorService executor = Executors.newFixedThreadPool(5);//creating a pool of 5 threads.
- for (int i = 0; i < 10; i++) {
- Runnable worker = new WorkerThread(“” + i);
How do you choose corePoolSize and maxPoolSize?
If the number of threads is equal (or greater than) the corePoolSize, put the task into the queue. If the queue is full, and the number of threads is less than the maxPoolSize, create a new thread to run tasks in. If the queue is full, and the number of threads is greater than or equal to maxPoolSize, reject the task.
How big is too big for the stack?
Stack overflow The stack has a limited size, and consequently can only hold a limited amount of information. On Windows, the default stack size is 1MB. On some unix machines, it can be as large as 8MB. If the program tries to put too much information on the stack, stack overflow will result.
How do you calculate stack capacity?
Stack capacity() method in Java with Example The capacity() method of Stack Class is used to get the capacity of this Stack. That is the number of elements present in this stack container. Parameters: This function accepts a parameter E obj which is the object to be added at the end of the Stack.