How do you change all caps to lowercase except first letter in Java?
Table of Contents
How do you change all caps to lowercase except first letter in Java?
toLowerCase(); Here substring(0,1). toUpperCase() convert first letter capital and substring(1). toLowercase() convert all remaining letter into a small case.
How do you make the first letter lowercase in Java?
What is the most efficient way to make the first character of a String lower case? String input = “SomeInputString”; char c[] = input. toCharArray(); c[0] = Character. toLowerCase(c[0]); String output = new String(c);
How do you capitalize the first letter of each word in a string in Java?

Java Program to capitalize each word in String
- public class StringFormatter {
- public static String capitalizeWord(String str){
- String words[]=str.split(“\\s”);
- String capitalizeWord=””;
- for(String w:words){
- String first=w.substring(0,1);
- String afterfirst=w.substring(1);
How do you lower the first letter of a string?
To capitalize the first letter of a string and lowercase the rest, use the charAt() method to access the character at index 0 and capitalize it using the toUpperCase() method. Then concatenate the result with using the slice() method to to lowercase the rest of the string.
How do I change all caps to lowercase except the first letter in word?
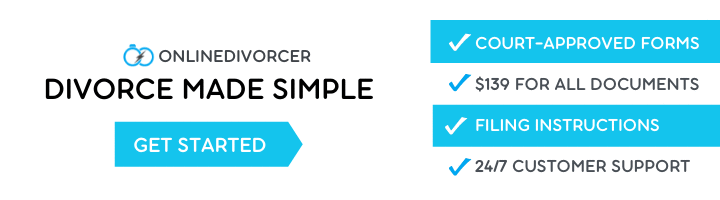
How to change uppercase and lowercase text in Microsoft Word
- Highlight all the text you want to change.
- Hold down the Shift and press F3 .
- When you hold Shift and press F3, the text toggles from sentence case (first letter uppercase and the rest lowercase), to all uppercase (all capital letters), and then all lowercase.
How do you change a character case in Java?
toUpperCase(char ch) converts the character argument to uppercase using case mapping information from the UnicodeData file. Note that Character. isUpperCase(Character.
How do you capitalize certain letters in Java?
The simplest way to capitalize the first letter of a string in Java is by using the String. substring() method: String str = “hello world!”; // capitalize first letter String output = str.
How can I capitalize the first letter of each word in a string?
To capitalize the first character of a string, We can use the charAt() to separate the first character and then use the toUpperCase() function to capitalize it.
How do you Decapitalize in Java?
Decapitalize a name. if (name. length() == 0) { return name; } else { String lowerName = name. toLowerCase(); int i; for (i = 0; i < name.
How do you Uncapitalize text?
To use a keyboard shortcut to change between lowercase, UPPERCASE, and Capitalize Each Word, select the text and press SHIFT + F3 until the case you want is applied.
How do you lowercase a string in Java?
Java String toLowerCase() Method The toLowerCase() method converts a string to lower case letters. Note: The toUpperCase() method converts a string to upper case letters.
How do you capitalize chars in Java?
Java Character toUpperCase() Method. The toUpperCase(char ch) method of Character class converts the given character argument to the uppercase using a case mapping information which is provided by the Unicode Data file. It should be noted that Character. isUpperase(Character.
Is there a nextChar in Java?
There is no classical nextChar() method in Java Scanner class. The best and most simple alternative to taking char input in Java would be the next().
How do I use charAt?
Counting Frequency of a character in a String by Using the charAt() Method
- public class CharAtExample5 {
- public static void main(String[] args) {
- String str = “Welcome to Javatpoint portal”;
- int count = 0;
- for (int i=0; i<=str.length()-1; i++) {
- if(str.charAt(i) == ‘t’) {
- count++;
- }
How do you change the case of a specific character in a string in Java?
To swap the case of a string, use.
- toLowerCase() for title case string.
- toLowerCase() for uppercase string.
- toUpperCase() for lowercase string.
How do you capitalize the first and last letter of a string in Java?
You simply need to take part after first and before last letter and add it to your upper-versions of first and last characters.