Is there Substr in C?
Table of Contents
Is there Substr in C?
Implement substr() function in C The substr() function returns the substring of a given string between two given indices. It returns the substring of the source string starting at the position m and ending at position n-1 .
How do you find a substring?
Simple Approach: The idea is to run a loop from start to end and for every index in the given string check whether the sub-string can be formed from that index. This can be done by running a nested loop traversing the given string and in that loop running another loop checking for sub-string from every index.
How do you check if a string contains a substring in c?

Check if String Contains Substring in C
- Use the strstr Function to Check if a String Contains a Substring in C.
- Use the strcasestr Function to Check if a String Contains a Substring.
- Use the strncpy Function to Copy a Substring.
What does * str ++ do in c?
To summarise, in the *str++ case you have an assignment, 2 increments, and a jump in each iteration of the loop. In the other case you only have 2 increments and a jump.
What is strstr () in C?
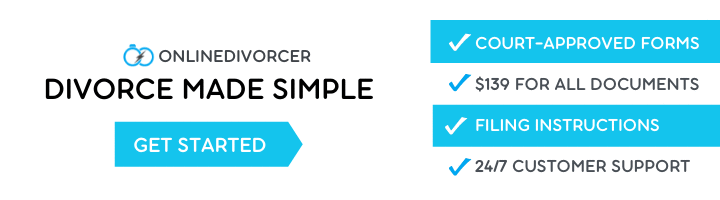
strstr() in C/C++ In C++, std::strstr() is a predefined function used for string handling. string. h is the header file required for string functions. This function takes two strings s1 and s2 as an argument and finds the first occurrence of the sub-string s2 in the string s1.
How do you find if a string contains a substring in C?
How do you find a substring in a string without using the library function in C++?
loc = search(source, target);
- if (loc == -1) printf(“\nNot found”);
- printf(“\nFound at location %d”, loc + 1); return (0);
- } int search(char src[], char str[]) {
- int i, j, firstOcc; i = 0, j = 0;
- while (src[i] != ‘\0’) {
- i++;
- return (-1);
- while (src[i] == str[j] && src[i] != ‘\0’
How do you check if a string contains a substring in C?
How do you split a string into parts based on a delimiter?
You can use the split() method of String class from JDK to split a String based on a delimiter e.g. splitting a comma-separated String on a comma, breaking a pipe-delimited String on a pipe, or splitting a pipe-delimited String on a pipe.