What does UnsupportedOperationException mean?
Table of Contents
What does UnsupportedOperationException mean?
a requested operation is not supported
An UnsupportedOperationException is a runtime exception in Java that occurs when a requested operation is not supported. For example, if an unmodifiable List is attempted to be modified by adding or removing elements, an UnsupportedOperationException is thrown.
Is UnsupportedOperationException checked or unchecked?
unchecked exception
It is an unchecked exception and thus, it does not need to be declared in a method’s or a constructor’s throws clause. Moreover, the UnsupportedOperationException exists since the 1.2 version of Java.
Is IllegalStateException a checked exception?
An IllegalStateException is an unchecked exception in Java. This exception may arise in our java program mostly if we are dealing with the collection framework of java. util.

What is a ClassCastException in Java?
ClassCastException is a runtime exception raised in Java when we try to improperly cast a class from one type to another. It’s thrown to indicate that the code has attempted to cast an object to a related class, but of which it is not an instance.
What is an IllegalArgumentException in Java?
An IllegalArgumentException is thrown in order to indicate that a method has been passed an illegal argument. This exception extends the RuntimeException class and thus, belongs to those exceptions that can be thrown during the operation of the Java Virtual Machine (JVM).
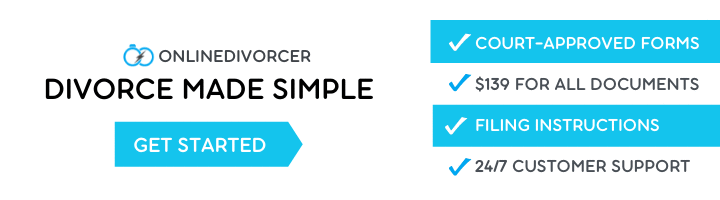
Which of the following is an example of runtime unchecked exception?
Java does not verify unchecked exceptions at compile-time. And although the above code does not have any errors during compile-time, it will throw ArithmeticException at runtime. Some common unchecked exceptions in Java are NullPointerException, ArrayIndexOutOfBoundsException and IllegalArgumentException.
What are exceptions six exception classes?
Common checked exceptions include IOException, DataAccessException, InterruptedException, etc. Common unchecked exceptions include ArithmeticException, InvalidClassException, NullPointerException, etc. 6. These exceptions are propagated using the throws keyword.
What is the difference between IllegalArgumentException and IllegalStateException?
The IllegalArgumentException is thrown in cases where the type is accepted but not the value, like expecting positive numbers and you give negative numbers. The IllegalStateException is thrown when a method is called when it shouldn’t, like calling a method from a dead thread.
When should you throw IllegalStateException?
This exception is thrown when you call a method at illegal or inappropriate time an IlleagalStateException is generated. For example, the remove() method of the ArrayList class removes the last element after calling the next() or previous methods.
Is ClassCastException checked or unchecked?
unchecked
ClassCastException is a subclass of the RuntimeException class which means it is an unchecked, runtime exception [4].
How do you solve ClassCastException?
// type cast an parent type to its child type. In order to deal with ClassCastException be careful that when you’re trying to typecast an object of a class into another class ensure that the new type belongs to one of its parent classes or do not try to typecast a parent object to its child type.
How do you throw an IllegalArgumentException?
For example, we can check an input parameter to our method and throw an IllegalArgumentException if it is invalid: public void calculate(int n) { if (n > MAX_VALUE) { throw new IllegalArgumentException(“Value too big (” + n + “)”); } }
Which of the following is an example of runtime unchecked exception Mcq?
Unchecked exception caught at run time when we execute the java program. Unchecked java exceptions example are ArithmeticException, null pointer exception etc. let’s say at the run time in the program if a number divide by zero occurs then arithmetic exception happens.
What are checked and unchecked exceptions examples?
Compare checked vs. unchecked exceptions
Criteria | Unchecked exception | Checked exception |
---|---|---|
List of examples | NullPointerException, ClassCastException, ArithmeticException, DateTimeException, ArrayStoreException | ClassNotFoundException, SocketException, SQLException, IOException, FileNotFoundException |
How many types of exceptions do we have?
An exception is an event which causes the program to be unable to flow in its intended execution. There are three types of exception—the checked exception, the error and the runtime exception.