How do I convert timestamp to date?
Table of Contents
How do I convert timestamp to date?
Let’s see the simple example to convert Timestamp to Date in java.
- import java.sql.Timestamp;
- import java.util.Date;
- public class TimestampToDateExample1 {
- public static void main(String args[]){
- Timestamp ts=new Timestamp(System.currentTimeMillis());
- Date date=new Date(ts.getTime());
- System.out.println(date);
- }
How do I change the format of a timestamp in SQL?
select to_char(sysdate, ‘YYYY-MM-DD’) from dual; To get this format by default, set it in your session’s NLS_DATE_FORMAT parameter: alter session set NLS_DATE_FORMAT = ‘YYYY-MM-DD’; You can also set the NLS_TIMESTAMP_FORMAT and NLS_TIMESTAMP_TZ_FORMAT .
How do I date a timestamp in SQL?
SQL Server comes with the following data types for storing a date or a date/time value in the database:

- DATE – format YYYY-MM-DD.
- DATETIME – format: YYYY-MM-DD HH:MI:SS.
- SMALLDATETIME – format: YYYY-MM-DD HH:MI:SS.
- TIMESTAMP – format: a unique number.
How do I format a specific date in SQL?
SQL Date Format with the FORMAT function
- Use the FORMAT function to format the date and time data types from a date column (date, datetime, datetime2, smalldatetime, datetimeoffset, etc.
- To get DD/MM/YYYY use SELECT FORMAT (getdate(), ‘dd/MM/yyyy ‘) as date.
How do I get timestamp from date and time?
You can simply use the fromtimestamp function from the DateTime module to get a date from a UNIX timestamp. This function takes the timestamp as input and returns the corresponding DateTime object to timestamp.
How do I format a date column in SQL?
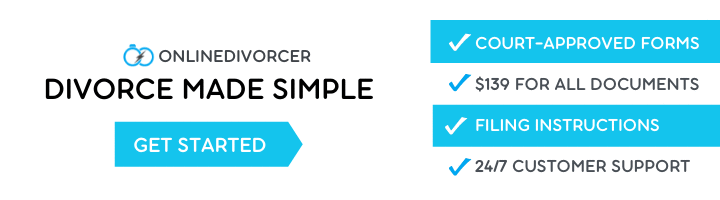
SQL Date Time Format Data Types
- DATE – format: YYYY-MM-DD.
- DATETIME – format: YYYY-MM-DD HH:MI:SS.
- TIMESTAMP – format: YYYY-MM-DD HH:MI:SS.
- YEAR – format YYYY or YY.
How convert datetime to DD MMM YYYY in SQL?
How do I get the date in YYYY-MM-DD format in SQL?
How to get different date formats in SQL Server
- Use the SELECT statement with CONVERT function and date format option for the date values needed.
- To get YYYY-MM-DD use this T-SQL syntax SELECT CONVERT(varchar, getdate(), 23)
- To get MM/DD/YY use this T-SQL syntax SELECT CONVERT(varchar, getdate(), 1)
What datatype is used for time in SQL?
Date and Time Data Types
Data type | Description | Storage |
---|---|---|
date | Store a date only. From January 1, 0001 to December 31, 9999 | 3 bytes |
time | Store a time only to an accuracy of 100 nanoseconds | 3-5 bytes |
datetimeoffset | The same as datetime2 with the addition of a time zone offset | 8-10 bytes |
What is timestamp in SQL?
MySQL TIMESTAMP() Function The TIMESTAMP() function returns a datetime value based on a date or datetime value. Note: If there are specified two arguments with this function, it first adds the second argument to the first, and then returns a datetime value.