Can you return an array in Java?
Table of Contents
Can you return an array in Java?
We can return an array in Java from a method in Java. Here we have a method createArray() from which we create an array dynamically by taking values from the user and return the created array.
How do you return an array element in Java?
get() is an inbuilt method in Java and is used to return the element at a given index from the specified Array. Parameters : This method accepts two mandatory parameters: array: The object array whose index is to be returned.
How do I return an array from a String?
Arrays. toString() method is used to return a string representation of the contents of the specified array. The string representation consists of a list of the array’s elements, enclosed in square brackets (“[]”). Adjacent elements are separated by the characters “, ” (a comma followed by a space).

How do you return a value in Java?
Let’s see a simple example to return integer value.
- public class ReturnExample1 {
- int display()
- {
- return 10;
- }
- public static void main(String[] args) {
- ReturnExample1 e =new ReturnExample1();
- System.out.println(e.display());
How do you return a string in Java?
- public static void main(String[] args) { // take input. Scanner scan = new Scanner(System.
- String str = scan. nextLine(); // reverse the string.
- // display result string. System. out.
- String rev = new String(); for(int i=s. length()-1; i>=0; i–){
- // On every iteration new string. // object will be created.
- } return rev;
Can a function return a function?
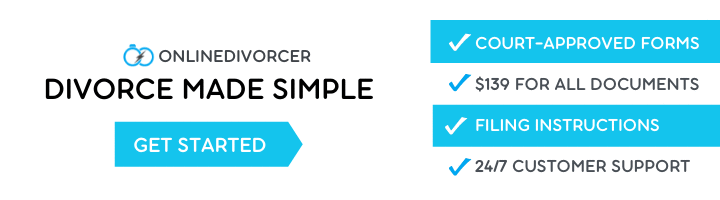
A function is an instance of the Object type. You can store the function in a variable. You can pass the function as a parameter to another function. You can return the function from a function.
How do you display an array in Java?
- public class Array { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; for (int element: array) { System.out.println(element); } } }
- import java.util.Arrays; public class Array { public static void main(String[] args) { int[] array = {1, 2, 3, 4, 5}; System.out.println(Arrays.toString(array)); } }
How do I print an array list?
These are the top three ways to print an ArrayList in Java:
- Using a for loop.
- Using a println command.
- Using the toString() implementation.
How do you access the values within an array?
You can use array subscript (or index) to access any element stored in array. Subscript starts with 0, which means arr[0] represents the first element in the array arr. In general arr[n-1] can be used to access nth element of an array.
What is the return type () method?
In computer programming, the return type (or result type) defines and constrains the data type of the value returned from a subroutine or method. In many programming languages (especially statically-typed programming languages such as C, C++, Java) the return type must be explicitly specified when declaring a function.
What is return in Java with example?
In Java, return is a reserved keyword i.e, we can’t use it as an identifier. It is used to exit from a method, with or without a value. Usage of return keyword as there exist two ways as listed below as follows: Case 1: Methods returning a value.
How do you return something in Java?
You declare a method’s return type in its method declaration. Within the body of the method, you use the return statement to return the value. Any method declared void doesn’t return a value. It does not need to contain a return statement, but it may do so.
How do I return a dynamic allocated array?
Return Dynamically Allocated Array Using Pointers Arrays can be returned by using dynamic allocation. Arrays can be dynamically allocated by using the word “new”. They will remain there until we delete them by ourselves. Static arrays are fixed in size, which means you have to provide size during initialization.
How do you pass an array to a method in Java?
To pass an array to a function, just pass the array as function’s parameter (as normal variables), and when we pass an array to a function as an argument, in actual the address of the array in the memory is passed, which is the reference.