Can we mock the constructor?
Table of Contents
Can we mock the constructor?
0, we can now mock Java constructors with Mockito. This allows us to return a mock from every object construction for testing purposes. Similar to mocking static method calls with Mockito, we can define the scope of when to return a mock from a Java constructor for a particular Java class.
Is there a default constructor in C++?
A default constructor is a constructor that either has no parameters, or if it has parameters, all the parameters have default values. If no user-defined constructor exists for a class A and one is needed, the compiler implicitly declares a default parameterless constructor A::A() .
What is NiceMock?
NiceMock is a subclass of MockFoo , so it can be used wherever MockFoo is accepted. It also works if MockFoo ‘s constructor takes some arguments, as NiceMock “inherits” MockFoo ‘s constructors: using ::testing::NiceMock; TEST(…)
How do you mock a function in C++?

It’s possible to use Google Mock to mock a free function (i.e. a C-style function or a static method). You just need to rewrite your code to use an interface (abstract class)….
- to define A* foo() in a separate source file foo. cpp in the main project,
- not to include foo.
- include a different source file mock-foo.
How do you mock a constructor method?
Faking static methods called in a constructor is possible like any other call. if your constructor is calling a method of its own class, you can fake the call using this API: // Create a mock for class MyClass (Foo is the method called in the constructor) Mock mock = MockManager. Mock(Constructor.
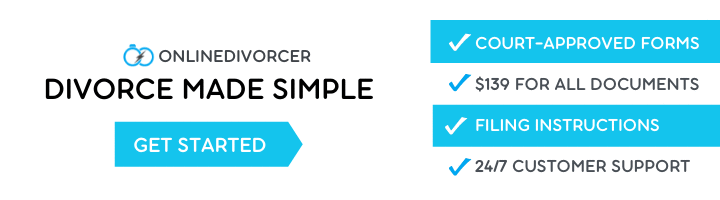
How do you create a default constructor in C++?
You can make your class default constructible (default in the first sense) by giving your constructor default arguments. struct S { S(int = 10) {} };
How do you call a default constructor?
You can just call default constructor with new operator (like this: new Test();) or this();. just Test() is forbidden because its not a method of class. Show activity on this post. In Java, the default constructor is the no-argument constructor that’s implicitly provided by the compiler.
What is Gtest and gMock?
Gtest is a framework for unit testing. Gmock is a framework imitating the rest of your system during unit tests. Follow this answer to receive notifications.
What is a strict mock?
To summarize, a strict mock will fail immediately if anything differs from the expectations. On the other hand, a non-strict mock (or a stub) will gladly “ignore” the call to foo. Quux and it should return a default(T) for the return type T of foo.
How do you stub a class in C++?
Creating Stub Implementation of Your Adaptor Class
- Add a C++ header file to the adaptor C++ project.
- Copy the following class definition into the header file.
- Add a C++ source file to the adaptor project.
- Copy the following stub implementations of all the adaptor virtual functions into the C++ source file.
How do you make a mock object?
Mock will be created by Mockito. Here we’ve added two mock method calls, add() and subtract(), to the mock object via when(). However during testing, we’ve called subtract() before calling add(). When we create a mock object using create(), the order of execution of the method does not matter.
Why do we use default constructor?
If not Java compiler provides a no-argument, default constructor on your behalf. This is a constructor initializes the variables of the class with their respective default values (i.e. null for objects, 0.0 for float and double, false for boolean, 0 for byte, short, int and, long).
What is the first statement in a default constructor?
The Eclipse compiler says, Constructor call must be the first statement in a constructor . So, it is not stopping you from executing logic before the call to super() . It is just stopping you from executing logic that you can’t fit into a single expression.