Can you split a dictionary Python?
Table of Contents
Can you split a dictionary Python?
Given a dictionary, the task is to split that dictionary into keys and values into different lists.
How do you split a key in Python?
To create a Python dictionary, we pass a sequence of items (entries) inside curly braces {} and separate them using a comma ( , ). Each entry consists of a key and a value, also known as a key-value pair.
Is slicing possible in dictionary?
Slicing a dictionary refers to obtaining a subset of key-value pairs present inside the dictionary. Generally, one would filter out values from a dictionary using a list of required keys. In this article, we will learn how to slice a dictionary using Python with the help of some relevant examples.

How will you get all the keys from the dictionary?
keys() method in Python Dictionary, returns a view object that displays a list of all the keys in the dictionary in order of insertion.
- Syntax: dict.keys()
- Parameters: There are no parameters.
- Returns: A view object is returned that displays all the keys.
Can we convert dictionary to list in Python?
Convert Dictionary Into a List of Tuples in Python. A dictionary can be converted into a List of Tuples using two ways. One is the items() function, and the other is the zip() function.
How does split function work in Python?
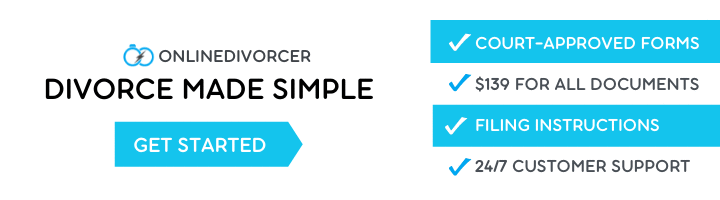
The split() function works by scanning the given string or line based on the separator passed as the parameter to the split() function. In case the separator is not passed as a parameter to the split() function, the white spaces in the given string or line are considered as the separator by the split() function.
How do you extract a value from a dictionary?
How to Extract Dictionary Values as a List
- (1) Using a list() function: my_list = list(my_dict.values())
- (2) Using a List Comprehension: my_list = [i for i in my_dict.values()]
- (3) Using For Loop: my_list = [] for i in my_dict.values(): my_list.append(i)
How do you slice a dictionary value in Python?
Given dictionary with value as lists, slice each list till K.
- Input : test_dict = {“Gfg” : [1, 6, 3, 5, 7], “Best” : [5, 4, 2, 8, 9], “is” : [4, 6, 8, 4, 2]}, K = 3.
- Output : {‘Gfg’: [1, 6, 3], ‘Best’: [5, 4, 2], ‘is’: [4, 6, 8]}
- Explanation : The extracted 3 length dictionary value list.
How do you filter a dictionary in Python?
Filter a Dictionary by values in Python using filter() filter() function iterates above all the elements in passed dict and filter elements based on condition passed as callback.
How do you extract information from a dictionary?
How do I get a list of keys in Python?
You can get all the keys in the dictionary as a Python List. dict. keys() returns an iterable of type dict_keys() . You can convert this into a list using list() .
How do I change a dictionary to a list?
- Given a dictionary, write a Python program to convert the given dictionary into list of tuples. Examples:
- Below are various methods to convert dictionary to list of tuples. Method #1 : Using list comprehension.
- Method #2 : Using items()
- Method #3 : Using zip.
- Method #4 : Using iteration.
- Method #5 : Using collection.
How do you turn a dictionary into a tuple?
Use the items() Function to Convert a Dictionary to a List of Tuples in Python. The items() function returns a view object with the dictionary’s key-value pairs as tuples in a list. We can use it with the list() function to get the final result as a list.
How do you split data in Python?
Scikit learn Split data
- x, y = num.
- array=() is used to define the array.
- list(y) is used to print the list of data on the screen.
- x_train, x_test, y_train, y_test = train_test_split(x, y, test_size=0.33, random_state=42) is used to split the dataframe into train and test data set.
How do I extract data from a dictionary in Python?
Here are 3 approaches to extract dictionary values as a list in Python:
- (1) Using a list() function: my_list = list(my_dict.values())
- (2) Using a List Comprehension: my_list = [i for i in my_dict.values()]
- (3) Using For Loop: my_list = [] for i in my_dict.values(): my_list.append(i)
How do you get a specific value out of a dictionary?
get() method is used in Python to retrieve a value from a dictionary. dict. get() returns None by default if the key you specify cannot be found. With this method, you can specify a second parameter that will return a custom default value if a key is not found.
How do you iterate through a nested dictionary in Python?
Iterate over all values of a nested dictionary in python For a normal dictionary, we can just call the items() function of dictionary to get an iterable sequence of all key-value pairs.
How do I filter a dictionary value?