How do I format a date in C++?
Table of Contents
How do I format a date in C++?
Date Formats Type
- MM/DD/YY: Month/Day/Year including leading zeros. (
- DD/MM/YY: Day/Month/Year including leading zeros. (
- YY/MM/DD: Year/Month/Day including leading zeros. (
- Month D, Y: Month name Day, Year including no leading zeros. (
- YYMMDD: YearMonthDate with no separators. (
How do I get the current date and time in C++?
3 Answers
- #include
- #include
- #include
- using namespace std;
- int main() {
- time_t timetoday;
- time(&timetoday);
- cout << “Calendar date and time as per todays is : ” << asctime(localtime(&timetoday));
How do I get CPP time?
Print system time in C++ (3 different ways)

- Using time() − It is used to find the current calendar time and have arithmetic data type that store time.
- localtime() − It is used to fill the structure with date and time.
- asctime() − It converts Local time into Human Readable Format.
How do you calculate time in a function in C++?
time() : time() function returns the time since the Epoch(jan 1 1970) in seconds. Prototype / Syntax : time_t time(time_t *tloc); Return Value : On success, the value of time in seconds since the Epoch is returned, on error -1 is returned.
What does time 0 do in C++?
The time(0) function returns the current time in seconds. The code that you upload will run all the code in one second. Therefore, even if the time is output, all the same time is output.
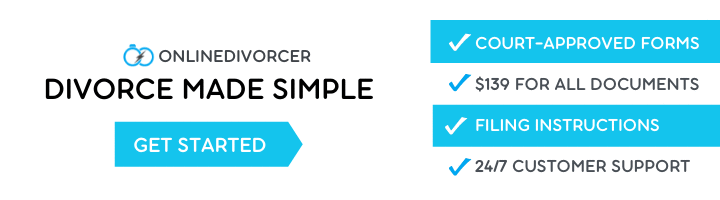
How do you create a timestamp in C++?
- int main()
- using namespace std::chrono;
- uint64_t ms = duration_cast(system_clock::now(). time_since_epoch()).
- std::cout << ms << ” milliseconds since the Epoch\n”;
- uint64_t sec = duration_cast(system_clock::now(). time_since_epoch()).
- std::cout << sec << ” seconds since the Epoch\n”;
- return 0;
Is there a date data type in C++?
The C++ standard library does not provide a proper date type. C++ inherits the structs and functions for date and time manipulation from C. To access date and time related functions and structures, you would need to include header file in your C++ program.
What is compile time in C++?
Compile-time is the time at which the source code is converted into an executable code while the run time is the time at which the executable code is started running.
What is format specifier in C++?
Format strings contain two types of objects: plain characters and format specifiers. Plain characters are copied verbatim to the resulting string. Format specifiers fetch arguments from the argument list and apply formatting to them.
What library is time in C++?
ctime header file
C++ time() The time() function in C++ returns the current calendar time as an object of type time_t . It is defined in the ctime header file.
How do I get UTC time in C++?
C++ gmtime() The gmtime() function in C++ converts the given time since epoch to calendar time which is expressed as UTC time rather than local time. The gmtime() is defined in header file.
What is data type in C++ with example?
Primitive Data Types: These data types are built-in or predefined data types and can be used directly by the user to declare variables. example: int, char, float, bool, etc. Primitive data types available in C++ are: Integer. Character.
What are the basic data types in C++?
C++ Fundamental Data Types
Data Type | Meaning | Size (in Bytes) |
---|---|---|
int | Integer | 2 or 4 |
float | Floating-point | 4 |
double | Double Floating-point | 8 |
char | Character | 1 |