How do you find all the methods of an object?
Table of Contents
How do you find all the methods of an object?
We are required to write a program (function) that takes in an object reference and returns an array of all the methods (member functions) that lives on that object. We are only required to return the methods in the array and not any other property that might have value of type other than a function. The Object.
How do you see all methods of an object in JS?
function getAllMethods(object) { return Object. getOwnPropertyNames(object). filter(function(property) { return typeof object[property] == ‘function’; }); } console. log(getAllMethods(Math));
Can JavaScript objects have methods?
Objects in JavaScript are collections of key/value pairs. The values can consist of properties and methods, and may contain all other JavaScript data types, such as strings, numbers, and Booleans.

Which is correct way to access method by the object?
To access the object, a method can use the this keyword. The value of this is the object “before dot”, the one used to call the method.
What are object methods in JavaScript?
JavaScript methods are actions that can be performed on objects. A JavaScript method is a property containing a function definition. Methods are functions stored as object properties.
How is an object property referenced in JavaScript?
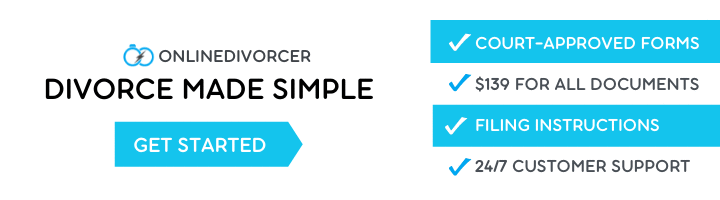
In JavaScript, objects are a reference type. Two distinct objects are never equal, even if they have the same properties. Only comparing the same object reference with itself yields true. For more information about comparison operators, see equality operators.
How do you print an object method?
To print all methods of an object, we can use Object. getOwnPropertyNames and check if the corresponding value is a function. The Object. getOwnPropertyNames() method returns an array of all properties (enumerable or not) found directly upon a given object.
What are properties and methods for objects in JavaScript?
Methods are actions that can be performed on objects. Object properties can be both primitive values, other objects, and functions. An object method is an object property containing a function definition. JavaScript objects are containers for named values, called properties and methods.
How do you associate function with object using JavaScript?
In JavaScript you can associate functions with objects. Here we will demonstrate how the constructor creates the object and assigns properties. Object is a standalone entity, with properties and type in JavaScript. JavaScript objects can have properties, that define their characteristics.
How do you access object objects?
You can access the properties of an object in JavaScript in 3 ways:
- Dot property accessor: object. property.
- Square brackets property access: object[‘property’]
- Object destructuring: const { property } = object.
How do you call a method using an object?
To call an object’s method, simply append the method name to an object reference with an intervening ‘. ‘ (period), and provide any arguments to the method within enclosing parentheses. If the method does not require any arguments, just use empty parentheses. objectReference.
Is JavaScript object pass by reference?
Objects and Arrays Objects in JavaScript are passed by reference. When more than one variable is set to store either an object , array or function , those variables will point to the same allocated space in the memory. Passed by reference.
How function is object in JavaScript?
In JavaScript, functions are first-class objects, because they can have properties and methods just like any other object. What distinguishes them from other objects is that functions can be called. In brief, they are Function objects. For more examples and explanations, see the JavaScript guide about functions.
How do you call a method from an object in Java?
Remember that.. The dot ( . ) is used to access the object’s attributes and methods. To call a method in Java, write the method name followed by a set of parentheses (), followed by a semicolon ( ; ). A class must have a matching filename ( Main and Main.
How to add a method to a JavaScript Object?
– Objects in JavaScript: Object Creation and Methods. In JS,objects are collections of key-value pairs.
How to know the type of an object in JavaScript?
Syntax. An expression representing the object or primitive whose type is to be returned.
What are the methods in JavaScript?
String Methods and Properties. Primitive values,like “John Doe”,cannot have properties or methods (because they are not objects).
How to get all property values of a JavaScript Object?
Dot property accessor: object.property