How do you sort files by size in Python?
Table of Contents
How do you sort files by size in Python?
Get list of files in directory sorted by size using glob()
- Get a list of all files in a directory using glob()
- Sort the list of files based on the size of files using sorted() function. For this, use os. stat(file_path). st_size to fetch the file size from stat object of file.
How do I sort a list of files in Python?
In Python, the os module provides a function listdir(dir_path), which returns a list of file and sub-directory names in the given directory path. Then using the filter() function create list of files only. Then sort this list of file names based on the name using the sorted() function.
How do I sort a list of files?
Sort Files and Folders

- In the desktop, click or tap the File Explorer button on the taskbar.
- Open the folder that contains the files you want to group.
- Click or tap the Sort by button on the View tab.
- Select a sort by option on the menu. Options.
How do I get the size of a file in Python?
Use the os. path. getsize(‘file_path’) function to check the file size. Pass the file name or file path to this function as an argument.
What is the sorted function in Python?
Python sorted() Function The sorted() function returns a sorted list of the specified iterable object. You can specify ascending or descending order. Strings are sorted alphabetically, and numbers are sorted numerically.
How do you sort by size?
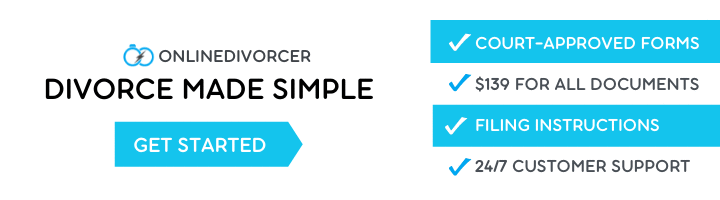
How do I sort all *. avi or *. py files in $HOME/Download/ directory by file size using Linux ls command line utility?…ls Command Sort Files By Size Command Options.
Option | Description |
---|---|
-l | Long listing |
-S | Sort by file size, largest first |
–sort=size | sort by size instead of file name(s) |
-r | Reverse order while sorting |
How do I list files in GB?
Using the ls Command
- –l – displays a list of files and directories in long format and shows the sizes in bytes.
- –h – scales file sizes and directory sizes into KB, MB, GB, or TB when the file or directory size is larger than 1024 bytes.
- –s – displays a list of the files and directories and shows the sizes in blocks.
How do you find the file size?
How to get file size in Java
- Files.size (NIO) This example uses NIO Files. size(path) to print the size of an image file (140 kb). GetFileSize.java.
- File. length (Legacy IO) In the old days (Before Java 7), we can use the legacy IO File. length() to get the size of a file in bytes.
What is the difference between sort and sorted in Python?
sort() function is very similar to sorted() but unlike sorted it returns nothing and makes changes to the original sequence. Moreover, sort() is a method of list class and can only be used with lists. Parameters: key: A function that serves as a key for the sort comparison.
How do you sort objects in Python?
How to sort the objects in a list in Python?
- my_list = [1, 5, 2, 6, 0] my_list.
- my_list = [1, 5, 2, 6, 0] print(sorted(my_list)) print(sorted(my_list, reverse=True))
- def get_my_key(obj): return obj[‘size’] my_list = [{‘name’: “foo”, ‘size’: 5}, {‘name’: “bar”, ‘size’: 3}, {‘name’: “baz”, ‘size’: 7}] my_list.
How do you create multiple files in Python with different names?
“how to create multiple files from one python file” Code Answer
- fn = open(“path of input file.txt”,”r”)
- for i, line in enumerate(fn):
- f = open(“/home/vidula/Desktop/project/ori_tri/input_%i.data” %i,’w’)
- f. write(line)
- f. close()
How do I sort ls by file size?
To sort the output of the ls command by file size, use the -l -s option followed by the -h option, which sorts files by human-readable file sizes (e.g. KB or MB).
Which option of ls sorts the files according to their size?
The -S option is the key, telling the ls command to sort the file listing by size.
How do you sort ls by size?
To list or sort all the files by size, use the -S option, that tells the ls command to sort the file listing by size and the -h option makes the output a human-readable format. In the following output, the largest files are shown in the beginning.
What is difference between readline and Readlines?
Python readline() method will return a line from the file when called. readlines() method will return all the lines in a file in the format of a list where each element is a line in the file.