Is there a non trivial destructor?
Table of Contents
Is there a non trivial destructor?
A class has a non-trivial destructor if it either has an explicitly defined destructor, or if it has a member object or a base class that has a non-trivial destructor. In example, I have a class, class C { public: ~C(); // not explicitly declared. };
Is destructor called automatically in C++?
A destructor is a member function that is invoked automatically when the object goes out of scope or is explicitly destroyed by a call to delete .
How do you define a deconstructor?
Destructor is an instance member function which is invoked automatically whenever an object is going to be destroyed. Meaning, a destructor is the last function that is going to be called before an object is destroyed. Destructor is also a special member function like constructor.

Can we have vitual destructor?
Yes, it is possible to have a pure virtual destructor. Pure virtual destructors are legal in standard C++ and one of the most important things to remember is that if a class contains a pure virtual destructor, it must provide a function body for the pure virtual destructor.
What is trivial destructor?
A trivial destructor is a destructor that performs no action. Objects with trivial destructors don’t require a delete-expression and may be disposed of by simply deallocating their storage. All data types compatible with the C language (POD types) are trivially destructible.
Is default destructor virtual?
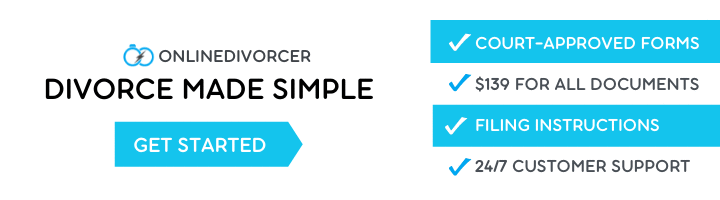
The destructor is not user-provided (meaning, it is either implicitly declared, or explicitly defined as defaulted on its first declaration) The destructor is not virtual (that is, the base class destructor is not virtual)
Can destructor be pure virtual?
What is a deconstructor in C++?
Destructors in C++ Destructors in C++ are members functions in a class that delete an object. They are called when the class object goes out of scope such as when the function ends, the program ends, a delete variable is called etc.
What is a trivial destructor?
What is destructor Oops?
In object-oriented programming, a destructor (sometimes abbreviated dtor) is a method which is invoked mechanically just before the memory of the object is released.
What are virtual destructors?
A virtual destructor is used to free up the memory space allocated by the derived class object or instance while deleting instances of the derived class using a base class pointer object.
Why do we need virtual destructors?
Virtual destructors in C++ are used to avoid memory leaks especially when your class contains unmanaged code, i.e., contains pointers or object handles to files, databases or other external objects. A destructor can be virtual.
Is implicit destructor virtual?
The implicitly-declared destructor is virtual (because the base class has a virtual destructor) and the lookup for the deallocation function (operator delete()) results in a call to ambiguous, deleted, or inaccessible function.
What is trivial type?
A trivial type is a type whose storage is contiguous (trivially copyable) and which only supports static default initialization (trivially default constructible), either cv-qualified or not. It includes scalar types, trivial classes and arrays of any such types.
What happens if destructor is not virtual?
Deleting a derived class object using a pointer of base class type that has a non-virtual destructor results in undefined behavior.
Can destructor be private?
Destructors with the access modifier as private are known as Private Destructors. Whenever we want to prevent the destruction of an object, we can make the destructor private.
Can constructor be virtual?
Constructor can not be virtual, because when constructor of a class is executed there is no vtable in the memory, means no virtual pointer defined yet.
How do you write a deconstructor in C++?
A destructor is a member function with the same name as its class prefixed by a ~ (tilde). For example: class X { public: // Constructor for class X X(); // Destructor for class X ~X(); }; A destructor takes no arguments and has no return type.