What is a Scanner class in Java?
Table of Contents
What is a Scanner class in Java?
The Scanner class is used to get user input, and it is found in the java.util package. To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation.
What is the Scanner class and why is it used?
Scanner is a class in java. util package used for obtaining the input of the primitive types like int, double, etc. and strings. It is the easiest way to read input in a Java program, though not very efficient if you want an input method for scenarios where time is a constraint like in competitive programming.
What are Scanner class methods?
Scanner Class Methods

Method | Description |
---|---|
nextFloat() | Reads a float value from the user |
nextInt() | Reads an int value from the user |
nextLine() | Reads a String value from the user |
nextLong() | Reads a long value from the user |
How do you write a Scanner class in Java?
Example 1
- import java.util.*;
- public class ScannerExample {
- public static void main(String args[]){
- Scanner in = new Scanner(System.in);
- System.out.print(“Enter your name: “);
- String name = in.nextLine();
- System.out.println(“Name is: ” + name);
- in.close();
How do you use a Scanner?
Just open the app, confirm what kind of content you want to capture — such as a document, a whiteboard, or a business card — and tap the shutter button. Microsoft Lens will handle the rest, including straightening out and neatly cropping your scan to make sure it looks proper and professional.
What is Scanner next ()?
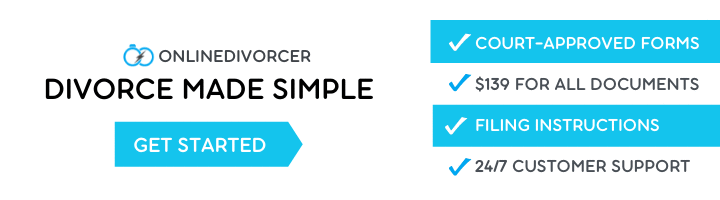
next() method finds and returns the next complete token from this scanner. A complete token is preceded and followed by input that matches the delimiter pattern. This method may block while waiting for input to scan, even if a previous invocation of hasNext() returned true.
Which is not a Scanner class method?
nextInt() Scanner class method is used to read integer value from the user. next() and nextLine() both methods can be used to read string from the user. nextSentence() is not used to scanner class. So, (d) nextSentence() is the correct answer.
How many methods are available in scanner class in java?
Java Scanner Methods to Take Input
Method | Description |
---|---|
nextBoolean() | reads a boolean value from the user |
nextLine() | reads a line of text from the user |
next() | reads a word from the user |
nextByte() | reads a byte value from the user |
How does scanner work in Java?
A simple text scanner which can parse primitive types and strings using regular expressions. A Scanner breaks its input into tokens using a delimiter pattern, which by default matches whitespace. The resulting tokens may then be converted into values of different types using the various next methods.
What are the advantages of scanner?
Scanners have many purposes which include copying, archiving, and sharing photos. The device captures images from print papers, photos, magazines, etc., and transfer them to the computer….Benefits of Scanning Documents
- Minimize paper storage.
- Increased security.
- Reduced costs.
- Environmentally friendly.
- Convenience.
How does scanner work java?
What is scanner next ()?
What is Scanner class constructor?
The Scanner class contains the constructors for specific purposes that we can use in our Java program. This constructor creates a Scanner object that produces values scanned from the specified file. This constructor constructs a new Scanner object that produces values scanned from the specified file.
What is token in Scanner class?
The tokens() method of Java Scanner class is used to get a stream of delimiter-separated tokens from the Scanner object which are in using. This method might block waiting for more input.
How does a Scanner work?
Scanners work by converting the image on the document into digital information that can be stored on a computer through optical character recognition (OCR). This process is done by a scanning head, which uses one or more sensors to capture the image as light or electrical charges.
What is Scanner close () in Java?
close() method closes this scanner. If this scanner has not yet been closed then if its underlying readable also implements the Closeable interface then the readable’s close method will be invoked. If this scanner is already closed then invoking this method will have no effect.