Does a JS class need a constructor?
Table of Contents
Does a JS class need a constructor?
Simple example no error if you don’t use a constructor in the class. Constructors require the use of the new operator to create a new instance, as such invoking a class without the new operator results in an error, as it’s required for the class constructor to create a new instance.
How do you initialize a class in JavaScript?
Classes are declared with the class keyword. We will use function expression syntax to initialize a function and class expression syntax to initialize a class. We can access the [[Prototype]] of an object using the Object. getPrototypeOf() method.
How can you declare a class with a constructor in JavaScript?
Class methods are created with the same syntax as object methods. Use the keyword class to create a class. Always add a constructor() method. Then add any number of methods.

What happens if you do not add a constructor to a class in JavaScript?
The constructor() method is called automatically when a class is initiated, and it has to have the exact name “constructor”, in fact, if you do not have a constructor method, JavaScript will add an invisible and empty constructor method.
Is constructor mandatory in class component?
No, you don’t need to.
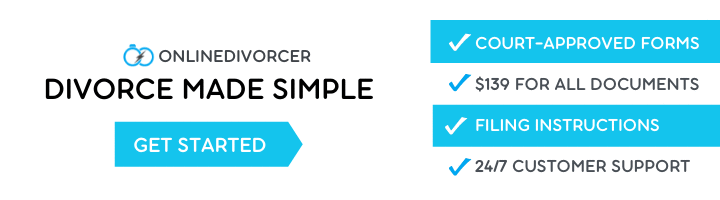
What happens if you do not add a constructor to a class?
If we don’t define a constructor in a class, then the compiler creates a default constructor(with no arguments) for the class.
How can we initialize an object in JavaScript?
Objects can be initialized using new Object() , Object. create() , or using the literal notation (initializer notation). An object initializer is a comma-delimited list of zero or more pairs of property names and associated values of an object, enclosed in curly braces ( {} ).
Which method of a class is called to initialize an object of that class in JavaScript?
The constructor method
The constructor method is a special method of a class for creating and initializing an object instance of that class.
How do we define a constructor when class name is?
To customize how a class initializes its members, or to invoke functions when an object of your class is created, define a constructor. A constructor has the same name as the class and no return value. You can define as many overloaded constructors as needed to customize initialization in various ways.
How are constructors used in class components?
We can use a constructor in the following ways:
- The constructor is used to initialize state. class App extends Component {
- Using ‘this’ inside constructor.
- Initializing third-party libraries.
- Binding some context(this) when you need a class method to be passed in props to children.
How do you initialize a state without a constructor?
Initialize State Without Constructor Another way of initializing state in React is to use the Class property. Once the class is instantiated in the memory all the properties of the class are created so that we can read these properties in the render function.
Does every class have a default constructor?
The compiler automatically provides a no-argument, default constructor for any class without constructors.
Is it always necessary to provide a default constructor for a class?
Java doesn’t require a constructor when we create a class. However, it’s important to know what happens under the hood when no constructors are explicitly defined. The compiler automatically provides a public no-argument constructor for any class without constructors.
What is initialize in JavaScript?
Initialization: When you declare a variable it is automatically initialized, which means memory is allocated for the variable by the JavaScript engine. Assignment: This is when a specific value is assigned to the variable.
How do you initialize a class in Java?
To initialize a class member variable, put the initialization code in a static initialization block, as the following section shows. To initialize an instance member variable, put the initialization code in a constructor.
Should class and constructor have same name?
The name of the constructor must be the same as the name of the class and, if you provide more than one constructor, the arguments to each constructor must differ in number or in type from the others. You do not specify a return value for a constructor.
Is constructor name is same as class name?
Every class object is created using the same new keyword, so it must have information about the class to which it must create an object. For this reason, the constructor name should be the same as the class name.
Can I use constructor in functional component?
In the case of class-based components, we can very easily use the constructor. When it comes to the functional components, we don’t have an easy way to use the constructor. But we can of course write custom hooks to achieve what the constructor does.