How do I enable scheduling in Spring?
Table of Contents
How do I enable scheduling in Spring?
The @EnableScheduling annotation is used to enable the scheduler for your application. This annotation should be added into the main Spring Boot application class file. The @Scheduled annotation is used to trigger the scheduler for a specific time period.
How do I schedule a batch file in Spring?
Spring Batch Scheduling: Spring Batch Jobs Scheduling You can configure Spring Batch Jobs in two different ways: Using the @EnableScheduling annotation. Creating a method annotated with @Scheduled and providing recurrence details with the job. Then add the job execution logic inside this method.
How does @scheduled work in Spring?
In addition to the TaskExecutor abstraction, Spring 3.0 introduces a TaskScheduler with a variety of methods for scheduling tasks to run at some point in the future. The simplest method is the one named ‘schedule’ that takes a Runnable and Date only. That will cause the task to run once after the specified time.

How do you dynamically schedule a spring batch job?
To configure, batch job scheduling is done in two steps:
- Enable scheduling with @EnableScheduling annotation.
- Create method annotated with @Scheduled and provide recurrence details using cron job. Add the job execution logic inside this method.
Where do I put EnableAsync?
The correct config would be: 1. @Service is above your MyService class; 2. @Async is above your method in MyService , 3. @EnableAsync , also with @SpringBootApplication be above your Application class.
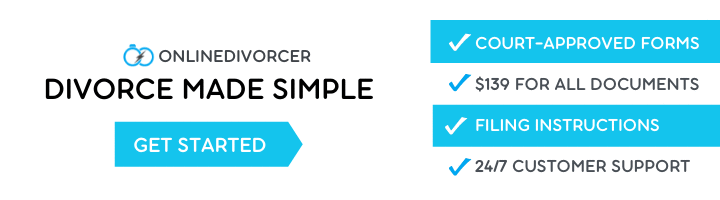
How do I run a Java scheduler?
How to run a task periodically in Java
- Scheduler Task. For this functionality, You should create a class extending TimerTask(available in java. util package).
- Run Scheduler Task. A class to run above scheduler task. Instantiate Timer Object Timer time = new Timer();
How do you schedule tasks?
How to Schedule Your Time
- Step 1: Identify Available Time. Start by establishing the time you want to make available for your work.
- Step 2: Schedule Essential Actions. Next, block in the actions you absolutely must take to do a good job.
- Step 3: Schedule High-Priority Activities.
- Step 4: Schedule Contingency Time.
How do I run a Spring Batch job continuously?
If you want to launch your jobs periodically, you can combine Spring Scheduler and Spring Batch. Here is a concrete example : Spring Scheduler + Batch Example. If you want to re-launch your job continually (Are you sure !), You can configure a Job Listener on your job. Then, through the method jobListener.
How do I turn on async in Spring?
To enable async configuration in spring, follow these steps:
- Create async thread pool. AsyncConfiguration.java. @Configuration.
- @Async controller methods. Methods which shall run asynchronously, annotate them with @Async annotation and method return type should return.
- Combine async method results. Inside REST Controller.
Is @EnableAsync mandatory?
It is mandatory to enable async support by annotating the main application class or any direct or indirect async method caller class with @EnableAsync annotation. By default mode is Proxy and another one is AspectJ.
What is task scheduling in Java?
Java programming language provides a class utility known as Timer Task. It allows one to schedule different tasks. In other words, a task can be executed after a given period or at a specified date and time. A Timer in Java is a process that enables threads to schedule tasks for later execution.
How do you schedule a task in Java?
One of the methods in the Timer class is the void schedule(Timertask task, Date time) method. This method schedules the specified task for execution at the specified time. If the time is in the past, it schedules the task for immediate execution.
How do you auto schedule tasks in MS Project?
On the Task tab, in the Schedule group, click Task Mode, and then click Auto Schedule. All new tasks entered in this project will have a default task mode of Automatically Scheduled.
How do I start and stop a scheduler in spring boot?
The schedulers do not start or stop. In the real world, it is necessary to stop and restart the scheduler without restarting the spring boot application. The ScheduledAnnotationBeanPostProcessor class allows you to programmatically start and stop the scheduler without having to restart the spring boot application.
What is enable async?
EnableAsync is used for configuration and enable Spring’s asynchronous method execution capability, it should not be put on your Service or Component class, it should be put on your Configuration class like: @Configuration @EnableAsync public class AppConfig { }
CAN REST API be asynchronous?
REST clients can be implemented either synchronously or asynchronously.
What does enable async do?
Annotation Type EnableAsync. Enables Spring’s asynchronous method execution capability, similar to functionality found in Spring’s XML namespace.
How do I schedule a Java program in TaskScheduler?
Run a java jar from Task Scheduler (Windows 7 Professional 64 bit)
- Step 1, install java, make sure it is available from commandline.
- Step 2, Open task scheduler:
- Step 3, make a new scheduled task:
- Step 4, configure your java to run:
- Step 5, Right click your new task for further configuration:
What is Spring Batch Scheduler?
In this tutorial we will be implementing Spring Boot Batch Scheduler with the help of example. Spring Batch is one of the open-source framework available for batch processing. Sometimes, while working on enterprise applications, we need to perform spring batch jobs from time to time or periodically on fixed time.