How do I find a specific text with beautiful soup?
Table of Contents
How do I find a specific text with beautiful soup?
Approach
- Import module.
- Pass the URL.
- Request page.
- Specify the tag to be searched.
- For Search by text inside tag we need to check condition to with help of string function.
- The string function will return the text inside a tag.
- When we will navigate tag then we will check the condition with the text.
- Return text.
How do you get a href value in BeautifulSoup?
To get href with Python BeautifulSoup, we can use the find_all method. to create soup object with BeautifulSoup class called with the html string. Then we find the a elements with the href attribute returned by calling find_all with ‘a’ and href set to True .
How do I find the HTML tag using python?
Approach:

- Import module.
- Scrap data from a webpage.
- Parse the string scraped to HTML.
- Use find() function to find the attribute and tag.
- Print the result.
What is Beautifulstonesoup?
Beautiful Soup is a Python library that is used for web scraping purposes to pull the data out of HTML and XML files. It creates a parse tree from page source code that can be used to extract data in a hierarchical and more readable manner.
How do I find text in HTML?
Use the textContent property to get the text of an html element, e.g. const text = box. textContent . The textContent property returns the text content of the element and its descendants. If the element is empty, an empty string is returned.
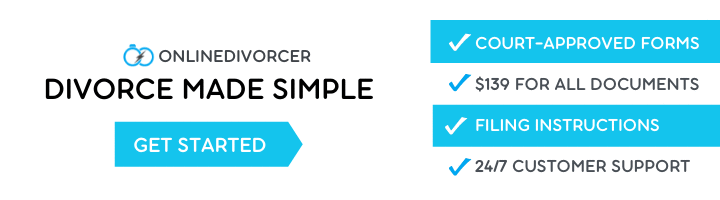
What is Find () method in BeautifulSoup?
The find method is used for finding out the first tag with the specified name or id and returning an object of type bs4. Example: For instance, consider this simple HTML webpage having different paragraph tags.
How do you extract href with beautiful soup?
How to get ‘href’ attribute of ‘a’ element using Beautiful Soup
- Then, import requests library.
- Get a source code of your target landing page.
- Convert HTML code into a Beautifulsoup object named soup.
- Then, find the href attribute you want to extract.
- Let’s check if our code works by printing it out.
- Results:
How do I extract links using BeautifulSoup?
Steps to be followed:
- Import the required libraries (bs4 and requests)
- Create a function to get the HTML document from the URL using requests.
- Create a Parse Tree object i.e. soup object using of BeautifulSoup() method, passing it HTML document extracted above and Python built-in HTML parser.
How do I find elements by class BeautifulSoup?
- Beautiful Soup has a built-in method that allows you to search for HTML tags by class name.
- Alternatively, you can search for HTML tags by class name using a CSS selector with BeautifulSoup select() method.
- Beautiful Soup allows you to use a function as a filter to search the HTML tree to find the elements you want.
What is the difference between FIND () and Find_all () in BeautifulSoup?
find is used for returning the result when the searched element is found on the page. find_all is used for returning all the matches after scanning the entire document.
What is LXML in BeautifulSoup?
To prevent users from having to choose their parser library in advance, lxml can interface to the parsing capabilities of BeautifulSoup through the lxml. html. soupparser module. It provides three main functions: fromstring() and parse() to parse a string or file using BeautifulSoup into an lxml.
How do I get text within an element?
Use the textContent property to get the text of a paragraph element, e.g. const result = p. textContent . The textContent property returns the text content of the p element and its descendants. If the element is empty, an empty string is returned.
How do I get text from an element?
Return the text content of an element:
- let text = element. textContent;
- element. textContent = “I have changed!”;
- let text = document. getElementById(“myList”). textContent;
How do you use BeautifulSoup to parse Google search results in Python?
Approach:
- Import the beautifulsoup and request libraries.
- Concatenate these two strings to get our search URL.
- Fetch the URL data using requests.
- Create a string and store the result of our fetched request, using request_result.
- Now we use BeautifulSoup to analyze the extracted page.
- We can do soup.