How do you search in binary?
Table of Contents
How do you search in binary?
Binary Search Working
- The array in which searching is to be performed is: Initial array.
- Set two pointers low and high at the lowest and the highest positions respectively.
- Find the middle element mid of the array ie.
- If x == mid, then return mid.
What is binary search C++?
Binary Search in C++ Binary Search is a method to find the required element in a sorted array by repeatedly halving the array and searching in the half. This method is done by starting with the whole array. Then it is halved.
What is binary search in Python?
Binary search is a searching algorithm which is used to search an element from a sorted array. It cannot be used to search from an unsorted array. Binary search is an efficient algorithm and is better than linear search in terms of time complexity. The time complexity of linear search is O(n).

Why is binary search used?
Binary search is a searching algorithm more efficient than linear search. It is used to find the position of an element in the array only if the array is sorted . It works on the principle of divide and conquer ie the technique repeatedly divides the array into halves.
How do I create a binary search in C++?
Binary search algorithm searches the target value within a sorted array.
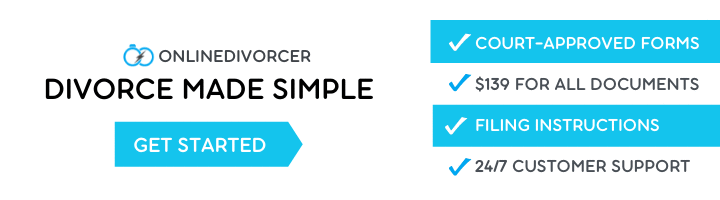
- To perform a binary search array must be sorted, it should either be in ascending or descending order.
- Step 1: First divide the list of elements in half.
- Step 2: In the second step we compare the target value with the middle element of the array.
How do you create a binary search tree in C++?
To create a BST in C++, we need to modify our TreeNode class in the preceding binary tree discussion, Building a binary tree ADT. We need to add the Parent properties so that we can track the parent of each node. It will make things easier for us when we traverse the tree.
How do binary searches work?
Binary search is an efficient algorithm for finding an item from a sorted list of items. It works by repeatedly dividing in half the portion of the list that could contain the item, until you’ve narrowed down the possible locations to just one.
Where is binary search used?
Binary search is used everywhere. Take any sorted collection from any language library (Java, . NET, C++ STL and so on) and they all will use (or have the option to use) binary search to find values.
Why do we use BST?
BSTs are used for a lot of applications due to its ordered structure. BSTs are used for indexing and multi-level indexing. They are also helpful to implement various searching algorithms. It is helpful in maintaining a sorted stream of data.
What are search trees used for?
In computer science, a search tree is a tree data structure used for locating specific keys from within a set. In order for a tree to function as a search tree, the key for each node must be greater than any keys in subtrees on the left, and less than any keys in subtrees on the right.
Where are binary search used?
How do you solve a binary search question?
Check for the peak at mid i.e. element at mid should be greater than element at (mid – 1) and (mid + 1). If yes, we’ve found the peak otherwise, check if element at (mid + 1) is greater than element at mid, if yes, move right else check if element at (mid -1) is greater than element at mid, if yes, move left.
Does C++ have built in binary search?
C++ bsearch() The bsearch() function in C++ performs a binary search of an element in an array of elements and returns a pointer to the element if found. The bsearch() function requires all elements less than the element to be searched to the left of it in the array.
How does the binary search algorithm work?
How do binary search trees work?
Definition. A binary search tree (BST) is a binary tree where each node has a Comparable key (and an associated value) and satisfies the restriction that the key in any node is larger than the keys in all nodes in that node’s left subtree and smaller than the keys in all nodes in that node’s right subtree.
Is binary search easy?
Binary search is faster than linear search except for small arrays. However, the array must be sorted first to be able to apply binary search. There are specialized data structures designed for fast searching, such as hash tables, that can be searched more efficiently than binary search.
Why binary search tree is faster?
The speed of deletion, insertion, and searching operations in Binary Tree is slower as compared to Binary Search Tree because it is unordered. Because the Binary Search Tree has ordered properties, it conducts element deletion, insertion, and searching faster.