How use hashCode and equals method in Java?
Table of Contents
How use hashCode and equals method in Java?
Contract for hashcode() method in Java If two objects are the same as per the equals(Object) method, then if we call the hashCode() method on each of the two objects, it must provide the same integer result.
How hashCode () and equals () methods are used in HashMap?
HashMap uses equals() to compare the key to whether they are equal or not. If the equals() method return true, they are equal otherwise not equal. A single bucket can have more than one node, it depends on the hashCode() method. The better your hashCode() method is, the better your buckets will be utilized.
What is the hashCode () and equals () function?
Java hashCode() An object hash code value can change in multiple executions of the same application. If two objects are equal according to equals() method, then their hash code must be same. If two objects are unequal according to equals() method, their hash code are not required to be different.

How override hashCode and equals method in Java with example?
Overriding hashCode method in Java
- Take a prime hash e.g. 5, 7, 17 or 31 (prime number as hash, results in distinct hashcode for distinct object)
- Take another prime as multiplier different than hash is good.
- Compute hashcode for each member and add them into final hash.
- Return hash.
What are equals () and hashCode () overriding rules?
if a class overrides equals, it must override hashCode. when they are both overridden, equals and hashCode must use the same set of fields. if two objects are equal, then their hashCode values must be equal as well. if the object is immutable, then hashCode is a candidate for caching and lazy initialization.
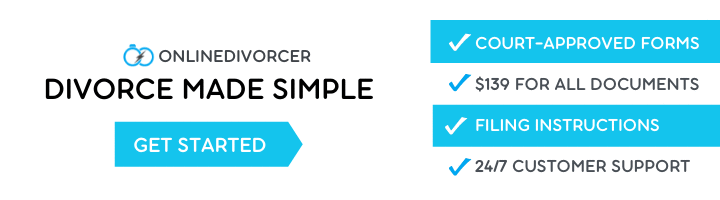
Why do we use equals and hashCode in Java?
Both methods, equals() and hashcode() , are used in Hashtable , for example, to store values as key-value pairs. If we override one and not the other, there is a possibility that the Hashtable may not work as we want, if we use such object as a key.
When should we override hashCode and equals?
Overriding only equals() method without overriding hashCode() causes the two equal instances to have unequal hash codes, which violates the hashCode contract (mentioned in Javadoc) that clearly says, if two objects are equal according to the equals(Object) method, then calling the hashCode method on each of the two …
Can two keys have same hashCode in Java?
It’s perfectly legal for two unequal objects to have the same hash code. It’s used by HashMap as a “first pass filter” so that the map can quickly find possible entries with the specified key. The keys with the same hash code are then tested for equality with the specified key.
How do you write equals method in Java?
Java String equals() Method Example 2
- public class EqualsExample2 {
- public static void main(String[] args) {
- String s1 = “javatpoint”;
- String s2 = “javatpoint”;
- String s3 = “Javatpoint”;
- System.out.println(s1.equals(s2)); // True because content is same.
- if (s1.equals(s3)) {
- System.out.println(“both strings are equal”);
Does Hashset use equals or hashCode?
The relation between equals and hashCode is: If equals returns true, then hashCode must return the same value. Otherwise a structure like java. util. HashSet won’t find the same bucket and could not guaranty that there are no two equal objects in a HashSet….
Equals and Hashcode | ||
---|---|---|
Prev | Chapter 6. Primary key mapping | Next |
Does HashSet use equals or hashCode?
What happens if we override hashCode and not equals?
Only Override HashCode, Use the default Equals: Only the references to the same object will return true. In other words, those objects you expected to be equal will not be equal by calling the equals method. Only Override Equals, Use the default HashCode: There might be duplicates in the HashMap or HashSet.
What happens if we don’t override hashCode and equals?
If you don’t override hashcode() then the default implementation in Object class will be used by collections. This implementation gives different values for different objects, even if they are equal according to the equals() method.
Can two hash codes be equal?
If two objects have the same hashcode then they are NOT necessarily equal. Otherwise you will have discovered the perfect hash function. But the opposite is true: if the objects are equal, then they must have the same hashcode .
What is equals in Java with example?
Definition and Usage The equals() method compares two strings, and returns true if the strings are equal, and false if not. Tip: Use the compareTo() method to compare two strings lexicographically.
Why we need to override both hashCode and equals method?
In order to use our own class objects as keys in collections like HashMap, Hashtable etc.. , we should override both methods ( hashCode() and equals() ) by having an awareness on internal working of collection. Otherwise, it leads to wrong results which we are not expected.