Can you use C library in C++?
Table of Contents
Can you use C library in C++?
Yes – C++ can use C libraries.
How do I link C and C++ files?
Just declare the C++ function extern “C” (in your C++ code) and call it (from your C or C++ code). For example: // C++ code: extern “C” void f(int);…For example:
- // C++ code:
- class C {
- // …
- virtual double f(int);
- };
- extern “C” double call_C_f(C* p, int i) // wrapper function.
- {
- return p->f(i);
How do you go from C to C++?
The three phases:

- Applying reusability. Write new code in C++ and link with existing C code.
- Develop Clean C. Modify existing C code to be acceptable to a C++ compiler.
- Use C+. Start using C++ language features to improve programming style, initially stopping short of using OOP features.
Is C++ backwards compatible with C?
C++ is not fully backward compatible with C, wherever it’s needed it has drawn a line.
How do third party libraries work in C++?
2 Answers
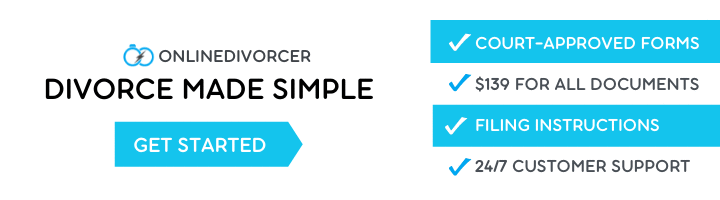
- Use the -I compiler option to point to the 3rd party libraries directory ( -I/usr/local/include/ohNet )
- Use #include “[whatever you need from oHNet].
- Use the -L linker option to specify a path to the 3rd party libs you need ( -L/usr/local/lib probably)
Should I learn C++ if I know C?
A great first step is to simply use C++ as “a better C,” which means that you can program in the C subset of C++ and find the experience better than in C because C++ provides extra type-checking and sometimes extra performance even for plain C code. Of course, C++ also provides much more!
Can C++ do everything C can?
works in C, but obviously can’t work in C++ because ‘new’ is a reserved word. There are some other ‘tricks’ with reserved words, but other than that, you can pretty much do everything in C that you can do in C++.
Is C++ fully backwards compatible with C?
How do I link an external library in Visual Studio code C++?
1 Answer
- install msys2 and then install SDL2 library using command on msys2 webpage.
- add bin folder of msys64(if your using 64 bit version) to system variables which has SDL dlls.
- include folder of msys64 contains header files of SDL2.
- lib folder contains libsdl.
How do I add external dependencies in Visual Studio C++?
Just right click on your project and select properties. There you will get another set of options under ‘Configuration Properties’ . Go to C/C++ and under that -> General -> Additional Include Directories ( where all the header files of third party is there ).
What are container Adaptors Mcq?
What are Container Adaptors? Explanation: Container Adaptors is the subset of Containers that provides a different interface for sequential containers.
How will you add a single line comment in C++?
Single-line comments start with two forward slashes ( // ). Any text between // and the end of the line is ignored by the compiler (will not be executed).