How do I convert a String to a date in Java?
Table of Contents
How do I convert a String to a date in Java?
Java String to Date
- import java.text.SimpleDateFormat;
- import java.util.Date;
- public class StringToDateExample1 {
- public static void main(String[] args)throws Exception {
- String sDate1=”31/12/1998″;
- Date date1=new SimpleDateFormat(“dd/MM/yyyy”).parse(sDate1);
- System.out.println(sDate1+”\t”+date1);
- }
How do I convert a String to a date?
How to convert a string to a date in Python
- from datetime import datetime.
-
- date_time_str = ’18/09/19 01:55:19′
-
- date_time_obj = datetime. strptime(date_time_str, ‘%d/%m/%y %H:%M:%S’)
-
-
- print (“The type of the date is now”, type(date_time_obj))
What is UTC date format in Java?
Parse String to ZonedDateTime in UTC Date time with full zone information can be represented in the following formats. dd/MM/uuuu’T’HH:mm:ss:SSSXXXXX pattern. e.g. “03/08/2019T16:20:17:717+05:30” . MM/dd/yyyy’T’HH:mm:ss:SSS z pattern.

How do I get GMT in Java?
Date date = new Date(); Calendar calendar = Calendar. getInstance(TimeZone. getTimeZone(“GMT”)); date1 = calendar. getTime();
What is the use of calendar getInstance () in java?
Calendar ‘s getInstance method returns a Calendar object whose calendar fields have been initialized with the current date and time: Calendar rightNow = Calendar.
How do you parse a UTC date string in Java?
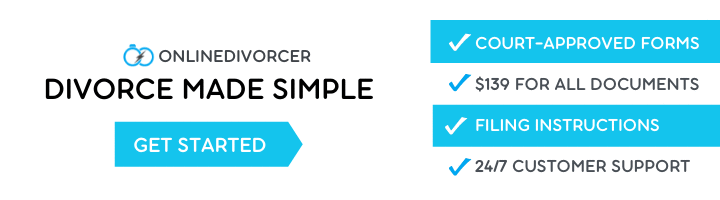
Method 1: Convert your UTC string to Instant. Using Instant you can create Date for any time-zone by providing time-zone string and use DateTimeFormatter to format date for display as you wish. LocalDate parsedDate = LocalDate. parse(dateString, DateTimeFormatter.
How do you convert a millisecond to a date in Java?
- Program to Convert Milliseconds to a Date Format in Java.
- Instant ofEpochMilli() method in Java with Examples.
- Instant parse() method in Java with Examples.
- Date toInstant() Method in Java with Examples.
- Date from() method in Java with Examples.
- Calendar Class in Java with examples.
How do I use calendar getInstance?
Example 1
- import java.util.Calendar;
- public class JavaCalendargetInstanceExample1 {
- public static void main(String args[]){
- Calendar mycal1 = Calendar.getInstance();
- Calendar mycal2 = Calendar.getInstance();
- mycal2.set(1996, 9 , 23);
- System.out.println(“mycal1 :” + mycal1.getTime());