How do I copy a binary search tree?
Table of Contents
How do I copy a binary search tree?
Algorithm to create a duplicate binary tree Create a new node and copy data of root node into new node. Recursively, create clone of left sub tree and make it left sub tree of new node. Recursively, create clone of right sub tree and make it right sub tree of new node. Return new node.
How do you copy a tree?
Accessing Copy Tree To perform a Copy Tree, open the PDM vault and navigate to the folder or top-level file you would like to copy, highlight it, select tools from the above toolbar, then select Copy Tree from the pop-out menu. The Copy Tree dialog box will then open, displaying the many options for your copy function.
What is a TreeNode Java?

public interface TreeNode. Defines the requirements for an object that can be used as a tree node in a JTree. Implementations of TreeNode that override equals will typically need to override hashCode as well. Refer to TreeModel for more information.
How do you copy a tree in data structure?
For copying, we have to do the following:
- create a node at copyTree , and the copy the value 2 into it.
- if orgTree->left != NULL , call copyInOrder( orgTree->left, copyTree->left );
- if orgTree->right != NULL , call copyInOrder( orgTree->right, copyTree->right );
Which traversal method to be used in cloning a tree?
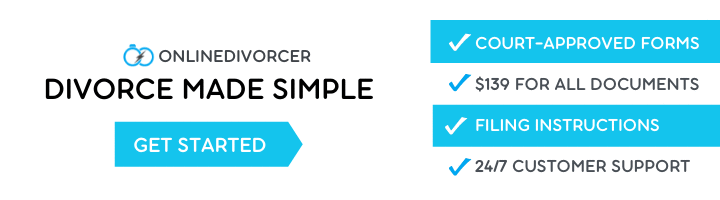
1) Recursively traverse the given Binary and copy key-value, left pointer, and a right pointer to clone tree. While copying, store the mapping from the given tree node to clone the tree node in a hashtable.
Can binary trees have duplicate values?
If you mean a “binary search tree”, my answer is “no”, since a search tree does not have any advantage in allowing duplicates, since SEARCHING for ONE value in a BST can only result in ONE value, not in two or more.
How do you recursively copy a binary tree?
The idea very simple – recursively traverse the binary tree in a preorder fashion, and for each encountered node, create a new node with the same data and insert a mapping from the original tree node to the new node in a hash table. After creating the mapping, recursively process its children.
What does cloning binary tree mean?
The idea is to store a mapping from given tree nodes to clone tree nodes in the hashtable. Following are detailed steps. 1) Recursively traverse the given Binary and copy key-value, left pointer, and a right pointer to clone tree.
What is difference between binary tree and binary search tree?
A Binary Tree is a non-linear data structure in which a node can have 0, 1 or 2 nodes. Individually, each node consists of a left pointer, right pointer and data element. A Binary Search Tree is an organized binary tree with a structured organization of nodes. Each subtree must also be of that particular structure.
What is a binary tree in Java?
A binary tree is a recursive data structure where each node can have 2 children at most. A common type of binary tree is a binary search tree, in which every node has a value that is greater than or equal to the node values in the left sub-tree, and less than or equal to the node values in the right sub-tree.
How do you traverse a general tree?
Preorder traversal of a general tree first visits the root of the tree, then performs a preorder traversal of each subtree from left to right. A postorder traversal of a general tree performs a postorder traversal of the root’s subtrees from left to right, then visits the root.
Can BST store duplicate values?
Of course yes. One node in BST has two children which are left child and right child. Left child has smaller value than parent node, meanwhile the right child has larger or equal value of parent node. Here is an example of BST with duplicate value.
How does binary search tree handle duplicates in Java?
So a Binary Search Tree by definition has distinct keys. How to allow duplicates where every insertion inserts one more key with a value and every deletion deletes one occurrence? A Better Solution is to augment every tree node to store count together with regular fields like key, left and right pointers.
What is copy binary tree?
Given a binary tree, efficiently create copy of it. Practice this problem. The idea very simple – recursively traverse the binary tree in a preorder fashion, and for each encountered node, create a new node with the same data and insert a mapping from the original tree node to the new node in a hash table.