How do you sort a list by string in Python?
Table of Contents
How do you sort a list by string in Python?
In Python, there are two ways, sort() and sorted() , to sort lists ( list ) in ascending or descending order. If you want to sort strings ( str ) or tuples ( tuple ), use sorted() .
Can a list of strings be sorted?
sort() list provides a member function sort(). It Sorts the elements of list in low to high order i.e. if list is of numbers then by default they will be sorted in increasing order. Whereas, if list is of strings then, it will sort them in alphabetical order.
How do you sort an array of strings alphabetically in Python?
Summary

- Use the Python List sort() method to sort a list in place.
- The sort() method sorts the string elements in alphabetical order and sorts the numeric elements from smallest to largest.
- Use the sort(reverse=True) to reverse the default sort order.
How do you sort a list of words in Python?
To sort a list alphabetically in Python, use the sorted() function. The sorted() function sorts the given iterable object in a specific order, which is either ascending or descending. The sorted(iterable, key=None) method takes an optional key that specifies how to sort.
In what order list of string can be?
A list of strings can be arranged in both ascending and descending order (option c).
How do you sort a list of strings in Python without sorting?
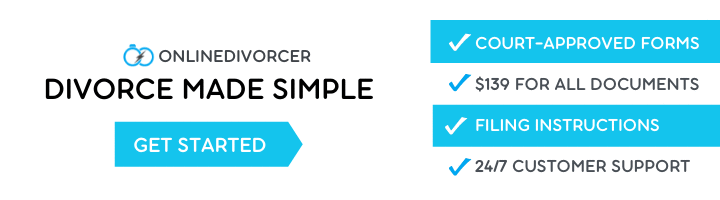
You can use Nested for loop with if statement to get the sort a list in Python without sort function. This is not the only way to do it, you can use your own logic to get it done.
How do you arrange a string in alphabetical order?
How to Sort a String in Java alphabetically in Java?
- Get the required string.
- Convert the given string to a character array using the toCharArray() method.
- Sort the obtained array using the sort() method of the Arrays class.
- Convert the sorted array to String by passing it to the constructor of the String array.
What is the difference between sorted and sort in Python?
In Python, sorting any sequence is very easy as it provides in-built methods for sorting. Two such methods are sorted() and sort()….Python3.
sorted() | sort() | |
---|---|---|
1. | The sorted() function returns a sorted list of the specific iterable object. | The sort() method sorts the list. |
How do you sort alphabets in Python?
Python Program to Sort Words in Alphabetic Order
- my_str = input(“Enter a string: “)
- # breakdown the string into a list of words.
- words = my_str. split()
- # sort the list.
- words. sort()
- # display the sorted words.
- for word in words:
- print(word)
How do you sort an array of strings alphabetically?
JavaScript Array sort() The sort() sorts the elements of an array. The sort() overwrites the original array. The sort() sorts the elements as strings in alphabetical and ascending order.
Which is better sort or sorted?
sort() is faster than sorted() because it doesn’t have to create a copy.
Which is faster sort or sorted in Python?
Sort vs Sorted Performance Here, sort() method is executing faster than sorted() function. Here, sorted() function method is executing faster than sort() function. There is also a difference in execution time in both cases.
How do you sort an array element in Python?
ALGORITHM:
- STEP 1: Declare and initialize an array.
- STEP 2: Loop through the array and select an element.
- STEP 3: The inner loop will be used to compare the selected element from the outer loop with the rest of the elements of the array.
- STEP 4: If any element is less than the selected element then swap the values.
How do you sort a string array using compareTo?
Sort a String Array Using the compareTo() Method in Java
- If string1 > string2 : return positive integer.
- If string1 < string2 : return negative integer.
- If string1 == string2 : return 0.
Which is faster sort or sorted Python?
What is difference between list sort and sorted in Python?
The primary difference between the two is that list. sort() will sort the list in-place, mutating its indexes and returning None , whereas sorted() will return a new sorted list leaving the original list unchanged.