How much memory should I allocate for a struct?
Table of Contents
How much memory should I allocate for a struct?
If we create an object of some structure, then the compiler allocates contiguous memory for the data members of the structure. The size of allocated memory is at least the sum of sizes of all data members. The compiler can use padding and in that case there will be unused space created between two data members.
How do I allocate dynamic memory to structure in CPP?
When you dynamically allocated memory for a struct you get a pointer to a struct. Once you are done with the Student you also have to remember to to release the dynamically allocated memory by doing a delete student1 . You can use a std::shared_ptr to manage dynamically allocated memory automatically.
How do I allocate more memory to C++?
You can allocate memory at run time within the heap for the variable of a given type using a special operator in C++ which returns the address of the space allocated. This operator is called new operator.

Do I need to allocate memory for struct in C?
Dynamic allocation of memory is a very important subject in C. It allows building complex data structures such as linked lists. Allocating memory dynamically helps us to store data without initially knowing the size of the data in the time we wrote the program.
How do you allocate memory in a struct?
Allocate Struct Memory With malloc in C
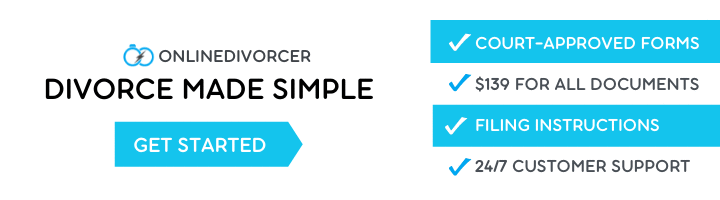
- Use malloc With the sizeof Operator to Allocate Struct Memory in C.
- Use the for Loop to Allocate Memory for an Array of Structs in C.
What section of memory will you allocate the struct instances in?
Structs are allocated on the stack, if a local function variable, or on the heap as part of a class if a class member.
How do you allocate and deallocate memory dynamically in C++?
In C++ when we want to allocate memory from the free-store (or we may call it heap) we use the new operator. int *ptr = new int; and to deallocate we use the delete operator.
How does C++ decide which memory to allocate data?
The two ways are: Compile time allocation or static allocation of memory: where the memory for named variables is allocated by the compiler. Exact size and storage must be known at compile time and for array declaration, the size has to be constant.
How do I dedicate more RAM to a program?
Allocate RAM Using Windows Task Manager
- On your Windows 10 PC, open the Windows Task Manager app. To do so, use your keyboard’s CTRL + SHIFT + ESC buttons.
- Go to the “Details Tab.”
- Right-click on the application you wish to assign extra RAM to and select priority.
How is memory allocated to variables declared in a structure?
- Memory is only allocated when you use the struct to declare variables or to dynamically allocate memory.
- There is not memory allocated on the heap, you have an automatic variable here.
- In your example, no memory is allocated, local variables that are NOT pointers are created on the stack.
Are structs stored in the heap?
Most importantly, a struct unlike a class, is a value type. So, while instances of a class are stored in the heap, instances of a struct are stored in the stack.
Does struct allocate memory?
This tells the compiler how big our struct is and how the different data items (“members”) are laid out in memory. But it does not allocate any memory. To allocate memory for a struct, we declare a variable using our new data type.
How is a struct stored in memory C++?
The members are stored in order, with padding inserted where necessary to correctly align each member relative to the start of the structure. Some compilers have a non-standard extension to “pack” the members, so that padding is not inserted.
How allocate memory dynamically in C++ explain with example?
Example 2: C++ new and delete Operator for Arrays Then, we have allocated the memory dynamically for the float array using new . We enter data into the array (and later print them) using pointer notation. After we no longer need the array, we deallocate the array memory using the code delete[] ptr; .
Does C++ need memory allocation?
In C++, we need to deallocate the dynamically allocated memory manually after we have no use for the variable. We can allocate and then deallocate memory dynamically using the new and delete operators respectively.
How do I allocate more virtual memory?
How to Increase Your Virtual Memory
- Head to Control Panel > System and Security > System.
- Select Advanced System Settings to open your System Properties. Now open the Advanced tab.
- Under Performance, select Settings. Open the Advanced tab. Under Virtual memory, select Change. Here are your Virtual Memory options.
How can I improve my committed memory?
How to Free Up RAM on Your Windows PC: 8 Methods
- Restart Your PC.
- Check RAM Usage With Windows Tools.
- Uninstall or Disable Unneeded Software.
- Update Your Apps.
- Use Lighter Apps and Manage Running Programs.
- Scan for Malware.
- Adjust Virtual Memory in Windows.
- Try ReadyBoost to Add More RAM.
Is memory allocated when a structure is declared?
Memory is allocated only when an instance of structure is created, till no memory is allocated. Here Structure reserved one bytes when it declare without any member declare inside it.
Where is struct stored in memory?
Memory allocation: Struct will be always allocated memory in Stack for all value types. Stack is a simple data structure with two operations i.e. Push and Pop . You can push on the end of the stack and pop of the end of stack by holding a pointer to the end of the Stack. All reference types will be stored in heap.