How do you write a Counter in Python?
Table of Contents
How do you write a Counter in Python?
c = Counter(x)
- c = Counter(x)
- Where x can be a list, dictionary, array, tuple or any other data structure available in Python.
What is Counter () Python?
Counter is a subclass of dict that’s specially designed for counting hashable objects in Python. It’s a dictionary that stores objects as keys and counts as values. To count with Counter , you typically provide a sequence or iterable of hashable objects as an argument to the class’s constructor.
How do you use the Counter method in Python?
Using the Python Counter tool, you can count the key-value pairs in an object, also called a hashtable object. The Counter holds the data in an unordered collection, just like hashtable objects. The elements here represent the keys and the count as values. It allows you to count the items in an iterable list.

How do you check the value of a Counter in Python?
To get the list of elements in the counter we can use the elements() method. It returns an iterator object for the values in the Counter.
How do you count objects in Python?
The count() function is used to count elements on a list as well as a string.
- Syntax: list_name.count(object)
- Parameters: The object is the things whose count is to be returned.
- Returns: count() method returns the count of how many times object occurs in the list.
- Exception:
How do you print a counter?
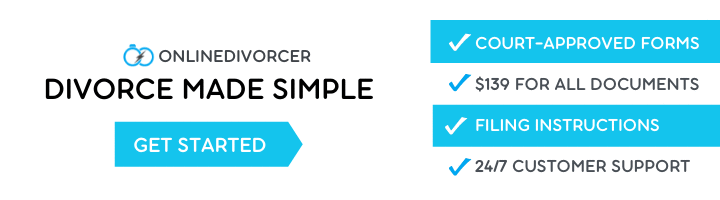
Checking the Print Counter
- Press the home button, if necessary.
- Select Settings.
- Select Print Counter. You see a screen like this:
- Select Print Sheet if you want to print out the counter results.
- Press the home button to exit.
How do you count characters in Python?
In Python, you can get the length of a string str (= number of characters) with the built-in function len() . This article describes the following contents.
What is counter method?
A Counter is a container that keeps track of how many times equivalent values are added. It can be used to implement the same algorithms for which bag or multiset data structures are commonly used in other languages.
How do you subtract two counters in Python?
Example 1:
- import collections. # create two boxes.
- box1 = collections.Counter({“pen”:4,”pencil”:6,”eraser”:3,”coins”:4}) box2 = collections.Counter({“pen”:2,”pencil”:2,”eraser”:1,”coins”:2})
- # subtract box2 from box1. ret = box1.subtract(box2)
- #print box1 contents. print(box1)
How do you define print in Python?
Python print() Function The print() function prints the specified message to the screen, or other standard output device. The message can be a string, or any other object, the object will be converted into a string before written to the screen.
Is Python counter sorted?
The official python document defines a counter as follows: “A Counter is a dict subclass for counting hashable objects. It is an unordered collection where elements are stored as dictionary keys and their counts are stored as dictionary values.
How do you count how many times something appears in a list in Python?
Using the count() Function The “standard” way (no external libraries) to get the count of word occurrences in a list is by using the list object’s count() function. The count() method is a built-in function that takes an element as its only argument and returns the number of times that element appears in the list.
How do you count inputs in Python?
Python String has got an in-built function – string. count() method to count the occurrence of a character or a substring in the particular input string. The string. count() method accepts a character or a substring as an argument and returns the number of times the input substring happens to appear in the string.
How do you add 1 to a counter in Python?
In python, if you want to increment a variable we can use “+=” or we can simply reassign it “x=x+1” to increment a variable value by 1. After writing the above code (python increment operators), Ones you will print “x” then the output will appear as a “ 21 ”. Here, the value of “x” is incremented by “1”.
How do you subtract a counter object value in Python?
Any element can easily be removed or deleted from a counter object by using =del=.
What is input () in Python?
In Python, we use input() function to take input from the user. Whatever you enter as input, the input function converts it into a string. If you enter an integer value still input() function convert it into a string.
How do you print multiple variables in Python?
To print multiple variables in Python, use the print() function. The print(*objects) is a built-in Python function that takes the *objects as multiple arguments to print each argument separated by a space.