How to get element by id a string?
Table of Contents
How to get element by id a string?
Use the document. querySelector() method to get an element by id by partially matching a string, e.g. const el = document. querySelector(‘[id*=”my-partial-id”]’) . The method returns the first element within the document that matches the provided selector.
How to get all id elements javascript?
Approach 2:
- First select all elements using $(‘*’) selector, Which selects every element of the Document.
- Use . map() method to traverse all element and check if it has ID.
- If it has ID then push it in the array using . get() method.
How do you select all elements with an ID?

The id selector uses the id attribute of an HTML element to select a specific element. The id of an element is unique within a page, so the id selector is used to select one unique element! To select an element with a specific id, write a hash (#) character, followed by the id of the element.
How do I get querySelectorAll ID?
Use the document. querySelectorAll() method to get all elements whose id starts with a specific string, e.g. document. querySelectorAll(‘[id^=”box”]’) . The method returns a NodeList containing all the elements that match the provided selector.
What is the use of get element by ID in JavaScript?
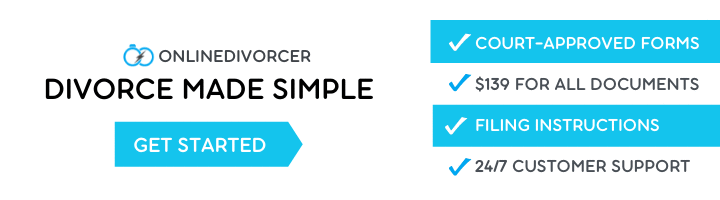
getElementById() The Document method getElementById() returns an Element object representing the element whose id property matches the specified string. Since element IDs are required to be unique if specified, they’re a useful way to get access to a specific element quickly.
What JavaScript method can be used to select an element based on its ID?
getElementById() method
In JavaScript, you can almost select any element from the DOM based on its unique ID by using the getElementById() method. It returns the first element that matches the given ID, or null if no matching element was found in the document.
How do I use querySelectorAll with ID?
Use the querySelectorAll() method to select elements by multiple ids, e.g. document. querySelectorAll(‘#box1, #box2, #box3’) . The method takes a string containing one or more selectors as a parameter and returns a collection of the matching elements.
What’s the use of getElementById () function?
How do I find element by id value?
The getElementById() method returns an element with a specified value. The getElementById() method returns null if the element does not exist. The getElementById() method is one of the most common methods in the HTML DOM. It is used almost every time you want to read or edit an HTML element.