How to give relative path in c# windows application?
Table of Contents
How to give relative path in c# windows application?
3 Answers
- string exeFile = (new System. Uri(Assembly. GetEntryAssembly(). CodeBase)). AbsolutePath; Once you have that, you can get it’s directory:
- string exeDir = Path. GetDirectoryName(exeFile); and turn your relative path to an absolute path:
- string fullPath = Path. Combine(exeDir, “.. \\.. \\Images\\Texture. dds”);
How to connect connection string in c#?
You can either use the new operator to make that directly. For example: SqlConnection conn = new SqlConnection( new SqlConnectionStringBuilder () { DataSource = “ServerName”, InitialCatalog = “DatabaseName”, UserID = “UserName”, Password = “UserPassword” }. ConnectionString );
How to write connection string in c# console application?
Connect to SQL Server in C# (example using Console application)
- “Server=localhost\sqlexpress”
- connetionString=”Data Source=IP_ADDRESS,PORT; Network Library=DBMSSOCN;Initial Catalog=DatabaseName; User ID=UserName;Password=Password”
- “Server= localhost; Database= databaseName; Integrated Security=SSPI;”
How to get connection string from SQL server c#?

C# SQL Server Connection
- Sql Server connection string. connetionString=”Data Source=ServerName; Initial Catalog=DatabaseName;User ID=UserName;Password=Password”
- Connect via an IP address.
- Trusted Connection from a CE device.
- Connecting to SQL Server using windows authentication.
How do I add a relative path in Visual Studio?
There are several options:
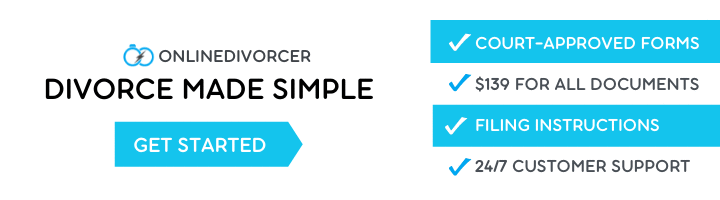
- Specifying a file or a path with nothing before it. For example: “mylib. lib”. In that case, the file will be searched at the Output Directory.
- If you add “.. \”, the path will be calculated from the actual path where the . sln file resides.
What is absolute path and relative path in C#?
In simple words, an absolute path refers to the same location in a file system relative to the root directory, whereas a relative path points to a specific location in a file system relative to the current directory you are working on.
What is ADO.NET connection string?
A connection string contains initialization information that is passed as a parameter from a data provider to a data source. The data provider receives the connection string as the value of the DbConnection. ConnectionString property.
What is ADO.NET connection?
An ADO.NET connection manager enables a package to access data sources by using a . NET provider. Typically, you use this connection manager to access data sources such as Microsoft SQL Server.
Where do we store connection string?
Connection strings can be stored as key/value pairs in the connectionStrings section of the configuration element of an application configuration file.
Where should you store the connection string information?
Connection strings in configuration files are typically stored inside the element in the app. config for a Windows application, or the web. config file for an ASP.NET application.
What is ADO.NET in C#?
ADO.NET is a set of classes that expose data access services for . NET Framework programmers. ADO.NET provides a rich set of components for creating distributed, data-sharing applications. It is an integral part of the . NET Framework, providing access to relational, XML, and application data.
What is a relative path in C#?
A relative rooted path specifies a drive, but its fully qualified path is resolved against the current directory. The following example illustrates the difference. C# Copy.
What is connection string in c# net?
Connection String is a normal String representation which contains Database connection information to establish the connection between Database and the Application.
How does connection string work?
In computing, a connection string is a string that specifies information about a data source and the means of connecting to it. It is passed in code to an underlying driver or provider in order to initiate the connection.