What is unsigned char* in c++?
Table of Contents
What is unsigned char* in c++?
unsigned char is a character datatype where the variable consumes all the 8 bits of the memory and there is no sign bit (which is there in signed char). So it means that the range of unsigned char data type ranges from 0 to 255.
When to use unsigned char?
It generally used to store character values. unsigned is a qualifier which is used to increase the values to be written in the memory blocks. For example – char can store values between -128 to +127, while an unsigned char can store value from 0 to 255 only.
Is char signed or unsigned?

The C and C++ standards allows the character type char to be signed or unsigned, depending on the platform and compiler. Most systems, including x86 GNU/Linux and Microsoft Windows, use signed char , but those based on PowerPC and ARM processors typically use unsigned char .
How do I print unsigned char pointer?
So when you are dealing with strings, you can always safely cast between unsigned char and char . (Pointer casts between the two types are formally poorly defined behavior by the standard, but in reality, it will always work on any system ever made.) Thus printf(“%s”, ptr); will work fine.
Why do you need signed and unsigned char?
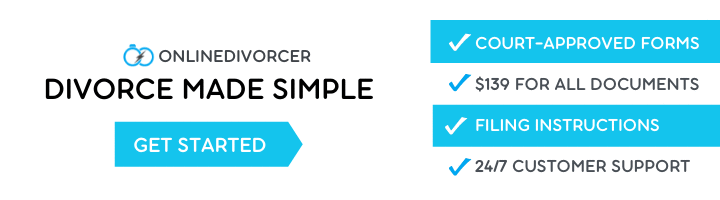
While the char data type is commonly used to represent a character (and that’s where it gets its name) it is also used when a very small amount of space, typically one byte, is needed to store a number. A signed char can store a number from -128 to 127, and an unsigned char can store a number from 0 to 255.
What is the difference between signed and unsigned char in C++?
An unsigned type can only represent postive values (and zero) where as a signed type can represent both positive and negative values (and zero). In the case of a 8-bit char this means that an unsigned char variable can hold a value in the range 0 to 255 while a signed char has the range -128 to 127.
Should I use uint8_t?
If the intended use of the variable is to hold an unsigned numerical value, use uint8_t; If the intended use of the variable is to hold a signed numerical value, use int8_t; If the intended use of the variable is to hold a printable character, use char.
Is byte same as unsigned char?
An unsigned char data type that occupies 1 byte of memory. It is the same as the byte datatype. The unsigned char datatype encodes numbers from 0 to 255. For consistency of Arduino programming style, the byte data type is to be preferred.
How do I print an unsigned char array?
What you need to do is print out the char values individually as hex values. printf(“hashedChars: “); for (int i = 0; i < 32; i++) { printf(“%x”, hashedChars[i]); } printf(“\n”); Since you are using C++ though you should consider using cout instead of printf (it’s more idiomatic for C++.