Can I mock a constructor?
Table of Contents
Can I mock a constructor?
0, we can now mock Java constructors with Mockito. This allows us to return a mock from every object construction for testing purposes. Similar to mocking static method calls with Mockito, we can define the scope of when to return a mock from a Java constructor for a particular Java class.
Can JUnit test class have constructor?
JUnit provides lot of methods to test our cases. We can test the constructor of a class using the same techniques we have used in our previous examples. Sometimes we need to initialize the objects and we do them in a constructor. In JUnit we can also do same using the @Before method.
How do you write a JUnit test case for a parameterized constructor in Java?

Steps to create a Parameterized JUnit test
- Step 1) Create a class.
- Step 2) Create a parameterized test class.
- @RunWith(class_name.
- Step 3) Create a constructor that stores the test data.
- Step 4) Create a static method that generates and returns test data.
How do you call a mock method in a constructor?
Faking static methods called in a constructor is possible like any other call. if your constructor is calling a method of its own class, you can fake the call using this API: // Create a mock for class MyClass (Foo is the method called in the constructor) Mock mock = MockManager. Mock(Constructor.
What is Jest fn ()?
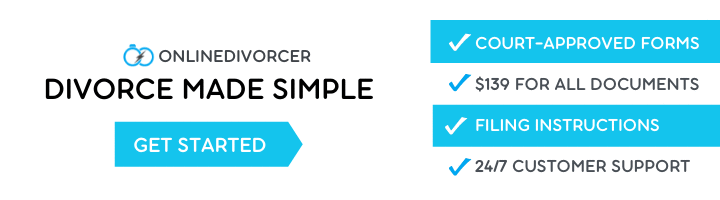
The jest. fn method is, by itself, a higher-order function. It’s a factory method that creates new, unused mock functions. Also, as we discussed previously, functions in JavaScript are first-class citizens. Each mock function has some special properties.
Do you test constructors?
To test that a constructor does its job (of making the class invariant true), you have to first use the constructor in creating a new object and then test that every field of the object has the correct value. Yes, you need need an assertEquals call for each field.
How do you test a constructor?
How do you write a test case for a parameterized constructor?
JUnit – Parameterized Test
- Annotate test class with @RunWith(Parameterized.
- Create a public static method annotated with @Parameters that returns a Collection of Objects (as Array) as test data set.
- Create a public constructor that takes in what is equivalent to one “row” of test data.
What is Jest testing?
Jest is an open-source testing framework built on JavaScript, designed majorly to work with React and React Native based web applications. Often, unit tests are not very useful when run on the frontend of any software. This is mostly because unit tests for the front-end require extensive, time-consuming configuration.
What is Jest FN mockImplementation?
mockImplementation(fn) Accepts a function that should be used as the implementation of the mock. The mock itself will still record all calls that go into and instances that come from itself – the only difference is that the implementation will also be executed when the mock is called.
How do you test a constructor in Java?
How do I test exceptions in a parameterized test?
Show activity on this post. if (parameter == EXCEPTION_EXPECTED) { try { method(parameter); fail(“didn’t throw an exception!”); } catch (ExpectedException ee) { // Test succeded! } }
What is stub in Java?
A stub is a controllable replacement for an existing dependency (or collaborator) in the system. By using a stub, you can test your code without dealing with the dependency directly. A mock object is a fake object in the system that decides whether the unit test has passed or failed.