Can you use stack for DFS?
Table of Contents
Can you use stack for DFS?
This recursive nature of DFS can be implemented using stacks. The basic idea is as follows: Pick a starting node and push all its adjacent nodes into a stack. Pop a node from stack to select the next node to visit and push all its adjacent nodes into a stack.
Does DFS use stack or queue?
BFS uses always queue, Dfs uses Stack data structure.
Can DFS be iterative?
Iterative Implementation of DFS It uses a stack instead of a queue. The DFS should mark discovered only after popping the vertex, not before pushing it. It uses a reverse iterator instead of an iterator to produce the same results as recursive DFS.

Is DFS recursive or iterative?
The only difference between iterative DFS and recursive DFS is that the recursive stack is replaced by a stack of nodes. Algorithm: Created a stack of nodes and visited array.
Is DFS LIFO or FIFO?
BFS is implemented using FIFO list on the other hand DFS is implemented using LIFO list.
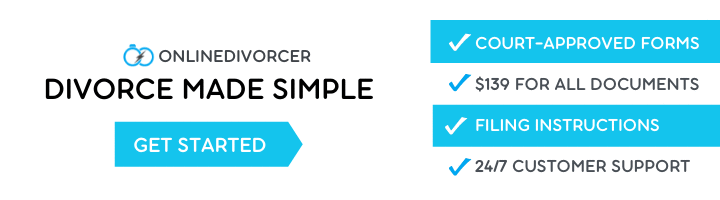
Why DFS is LIFO?
We use the LIFO queue, i.e. stack, for implementation of the depth-first search algorithm because depth-first search always expands the deepest node in the current frontier of the search tree. The search proceeds immediately to the deepest level of the search tree, where the nodes have no successors.
Why stack is used in DFS?
Depth First Search (DFS) algorithm traverses a graph in a depthward motion and uses a stack to remember to get the next vertex to start a search, when a dead end occurs in any iteration.
Is iterative DFS faster than recursive?
They both work fine on files with small sizes (less than 1 MB). However, when I try to run them over files with 50 MB, it seems like that the recursive-DFS (9 secs) is much faster than that using an iterative approach (at least several minutes). In fact, the iterative approach took ages to finish.
Can you implement BFS using stack?
Introduction. This article describes breadth-first search (BFS) on a tree (level-wise search) using a stack, either using a procedure allocated stack or using a separate stack data structure. BFS is a way of scanning a tree while breadth is performed first.
Can you do DFS on a directed graph?
Find the strong components of a directed graph. If the graph has n vertices and m edges then depth first search can be used to solve all of these problems in time O(n + m), that is, linear in the size of the graph.
Does DFS use FIFO?
DFS traverses according to tree depth. It is implemented using FIFO list.
Is a stack FIFO?
Stack is a LIFO (last in first out) data structure. The associated link to wikipedia contains detailed description and examples. Queue is a FIFO (first in first out) data structure.
What is stacked bar chart?
A stacked bar chart is a type of bar graph that represents the proportional contribution of individual data points in comparison to a total. The height or length of each bar represents how much each group contributes to the total.
What are the 3 things a graph must have?
Essential Elements of Good Graphs:
- A title which describes the experiment.
- The graph should fill the space allotted for the graph.
- Each axis should be labeled with the quantity being measured and the units of measurement.
- Each data point should be plotted in the proper position.
- A line of best fit.
How do you choose the best scale for a graph?
I like to give students the following tips when demonstrating selecting an appropriate scale:
- Try to make the graph fill most of the space, using a break in the axis scale if appropriate.
- To make the divisions on an axis easy to plot, factors of two or five are probably best.
Why DFS is faster than BFS?
DFS is faster than BFS. Time Complexity of BFS = O(V+E) where V is vertices and E is edges. Time Complexity of DFS is also O(V+E) where V is vertices and E is edges.