How do you sort a linked list using bubble sort?
Table of Contents
How do you sort a linked list using bubble sort?
Given a singly linked list, sort it using bubble sort by swapping nodes….Approach:
- Get the Linked List to be sorted.
- Apply Bubble Sort to this linked list, in which, while comparing the two adjacent nodes, actual nodes are swapped instead of just swapping the data.
- Print the sorted list.
Is bubble sort suitable for linked list?
Bubble sort is just as efficient (or rather inefficient) on linked lists. We can easily bubble sort even a singly linked list.
How do you sort a linked list using bubble sort in C++?
To perform bubble sort, we follow below steps:

- Step 1: Check if data on the 2 adjacent nodes are in ascending order or not. If not, swap the data of the 2 adjacent nodes.
- Step 2: At the end of pass 1, the largest element will be at the end of the list.
- Step 3: We terminate the loop, when all the elements are started.
How do you sort data in a linked list?
Below is a simple insertion sort algorithm for a linked list. 1) Create an empty sorted (or result) list 2) Traverse the given list, do following for every node. ……a) Insert current node in sorted way in sorted or result list. 3) Change head of given linked list to head of sorted (or result) list.
What is bubble sorting in C?
Bubble sort in C is a straightforward sorting algorithm that checks and swaps elements if they are not in the intended order. It compares two adjacent elements to find which one is greater or lesser and switches them based on the given condition until the final place of the element is found.
How do you write a bubble sort in C?
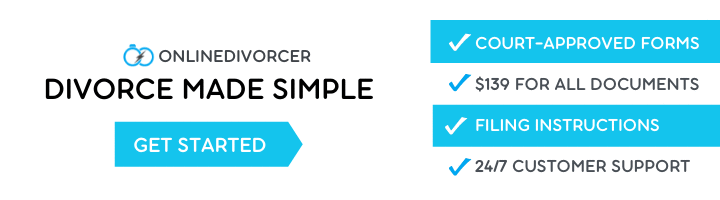
Working of Bubble Sort in C
- Starts from the first index: arr[0] and compares the first and second element: arr[0] and arr[1]
- If arr[0] is greater than arr[1], they are swapped.
- Similarly, if arr[1] is greater than arr[2], they are swapped.
- The above process continues until the last element arr[n-1]
What is sorting bubble sort?
Bubble sort, sometimes referred to as sinking sort, is a simple sorting algorithm that repeatedly steps through the list, compares adjacent elements and swaps them if they are in the wrong order. The pass through the list is repeated until the list is sorted.
How do you implement bubble sort?
Working of Bubble Sort
- Starting from the first index, compare the first and the second elements.
- If the first element is greater than the second element, they are swapped.
- Now, compare the second and the third elements. Swap them if they are not in order.
- The above process goes on until the last element.