Can array have NULL values JavaScript?
Table of Contents
Can array have NULL values JavaScript?
To check if all of the values in an array are equal to null , use the every() method to iterate over the array and compare each value to null , e.g. arr. every(value => value === null) . The every method will return true if all values in the array are equal to null .
How do you check the array is empty or not in JavaScript?
The array can be checked if it is empty by using the array. length property. This property returns the number of elements in the array. If the number is greater than 0, it evaluates to true.
How do you filter null in array?
To remove all null values from an array:

- Call the filter() method, passing it a function.
- The function should check whether each array element is not equal to null .
- The filter method returns a new array, containing only the elements that satisfy the condition.
How do I check if an array is empty in typescript?
How to check array is empty in typescript?
- const users: Array = [];
- if(typeof users !== “undefined” && users. length == 0){
- console. log(“Array is empty”);
Can you put null into an array?
An array value can be non-empty, empty (cardinality zero), or null. The individual elements in the array can be null or not null.
Can we add null in array?
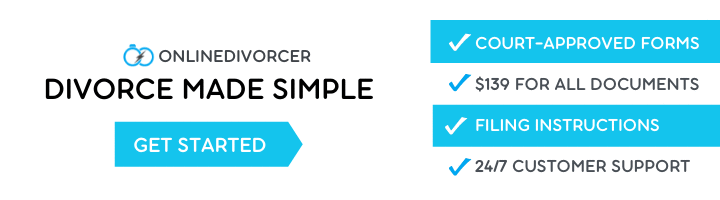
Yes, you can always use null instead of an object.
How do you check if an element in an array is null?
To check if an array is null, use equal to operator and check if array is equal to the value null. In the following example, we will initialize an integer array with null. And then use equal to comparison operator in an If Else statement to check if array is null. The array is empty.
Is an empty array null?
An empty array, an array value of null, and an array for which all elements are the null value are different from each other. An uninitialized array is a null array.
Why is my JavaScript array undefined?
You get undefined when you try to access the array value at index 0, but it’s not that the value undefined is stored at index 0, it’s that the default behavior in JavaScript is to return undefined if you try to access the value of an object for a key that does not exist.
How do I add an element to a null array in Java?
“java can you add elements to an empty array” Code Answer
- int[] nums = new int[5];
- for(int i = 0; i < nums. length; i++){
- nums[i] = i + 2;
- System. out. println(nums[i]);
- }
- /*
- OUTPUT:
- 2 3 4 5 6.
Can int array contain null?
int is a primitive type in java, and cannot be null. Only objects can be null.
How do you check if an array is null?
Can you have a null array?
How do you remove the null from an object array?
To remove all null values from an object:
- Call the Object. keys() method to get an array of the object’s keys.
- Call the forEach() method to iterate over the array and delete all null values using the delete operator.
How do you write null in JavaScript?
In JavaScript, null is a special value that represents an empty or unknown value. For example, let number = null; The code above suggests that the number variable is empty at the moment and may have a value later.
How do you add items to an empty array?
Answer: Use the array_push() Function You can simply use the array_push() function to add new elements or values to an empty PHP array.
How do you add something to an empty array?
To add elements to an empty array, we use the indexer to specify the element and assign a value to it with the assignment operator. Unfortunately, there’s no shortcut to add elements to an array dynamically. If we want to do that, we would have to use another collection type like an ArrayList .
How to check object is null or empty in JavaScript?
Answer: Use the Object. keys() Method obj != null will catch both null and undefined values. The isEmptyObject() function in the following example will check if an object is empty. However, it will only test plain objects (created using ” {} ” or ” new Object() “).