How do I convert a string to a double in C++?
Table of Contents
How do I convert a string to a double in C++?
C++ string to float and double Conversion The easiest way to convert a string to a floating-point number is by using these C++11 functions: std::stof() – convert string to float. std::stod() – convert string to double. std::stold() – convert string to long double .
How do I turn off scientific notation in C++?
you need to write: cout<< fixed; cout<< setprecision(2)<< f; fixed disables the scientific notation i.e. 1.23e+006…. and fixed is a sticky manipulator so u need to disable it if u want to revert back to scientific notation…
Does C++ recognize scientific notation?

Finally, this notation always includes an exponential part consisting on the letter e followed by an optional sign and three exponential digits. write floating-point values in scientific notation….std::scientific.
flag value | effect when set |
---|---|
scientific | write floating-point values in scientific notation. |
How do you express scientific notation in C++?
Because it can be hard to type or display exponents in C++, we use the letter ‘e’ (or sometimes ‘E’) to represent the “times 10 to the power of” part of the equation. For example, 1.2 x 10⁴ would be written as 1.2e4 , and 5.9736 x 10²⁴ would be written as 5.9736e24 .
What does stod mean in C++?
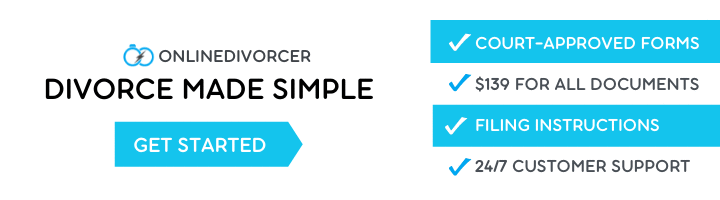
String object with the representation of a floating-point number. idx. Pointer to an object of type size_t, whose value is set by the function to position of the next character in str after the numerical value. This parameter can also be a null pointer, in which case it is not used.
What is 1e9 in C++?
1e-9 is 0.000000001 ; the minus sign applies to the exponent. -1e9 is -1000000000.0 ; the minus sign applies to the number itself. The e (or E ) means “times 10-to-the”, so 1e9 is “one times ten to the ninth power”, and 1e-9 means “one times ten to the negative ninth power”.
What is 1e5 in C++?
so 1e5 is equal to 100000 , both notations are interchangeably meaning the same.
Which method is used to convert a string to double?
Syntax: double str1 = Double. parseDouble(str);
What is double parseDouble?
Double parseDouble() method in Java with examples The parseDouble() method of Java Double class is a built in method in Java that returns a new double initialized to the value represented by the specified String, as done by the valueOf method of class Double.
What does STOF mean in C++?
std::stof in C++ Parses string interpreting its content as a floating-point number, which is returned as a value of type float.
Is 1e9 double?
1e9 is a double that has an exact representation in the IEEE floating point representation. The C++ standard doesn’t mandate IEEE floating point. s is an int , so the double value will be automatically converted to an int .
IS 10/9 the same as 1e9?
Actually, dbaupp is correct. The e1 parts means *10^9. Nope, 1e9 = 1 * 10^9.
What is 1E10 in C++?
The value of 1E10 when assigned to an integer is “1410065408” for MSVC compiler and whereas for intel C++ is “-2147483648”.
Is 10e6 the same as 1e7?
And that’s what I was talking about from the start—10e6 is 10×10⁶, which is in normalised form 1×10⁷ or 1e7.
How do I print double in C++?
We can print the double value using both %f and %lf format specifier because printf treats both float and double are same. So, we can use both %f and %lf to print a double value.
Can we convert string to double?
We can convert String to Double object through it’s constructor too. Also if we want double primitive type, then we can use doubleValue() method on it. Note that this constructor has been deprecated in Java 9, preferred approach is to use parseDouble() or valueOf() methods.
Can you cast a string to a double?
We can convert String to double in java using Double. parseDouble() method.