How do you check if an item already exists in a list in C#?
Table of Contents
How do you check if an item already exists in a list in C#?
public bool Contains (T item); Here, item is the object which is to be locate in the List. The value can be null for reference types. Return Value: This method returns True if the item is found in the List otherwise returns False.
How do you check if a string exists in a list C#?
if (myList. Contains(myString)) string element = myList. ElementAt(myList. IndexOf(myString));
What is all in Linq C#?
The Linq All Operator in C# is used to check whether all the elements of a data source satisfy a given condition or not. If all the elements satisfy the condition, then it returns true else return false. There is no overloaded version is available for the All method.

Where with Contains in Linq?
LINQ Contains operator is used to check whether an element is available in sequence (collection) or not. Contains operator comes under Quantifier Operators category in LINQ Query Operators. Below is the syntax of Contains operator.
What is IEquatable in C#?
The IEquatable interface is used by generic collection objects such as Dictionary, List, and LinkedList when testing for equality in such methods as Contains , IndexOf , LastIndexOf , and Remove . It should be implemented for any object that might be stored in a generic collection.
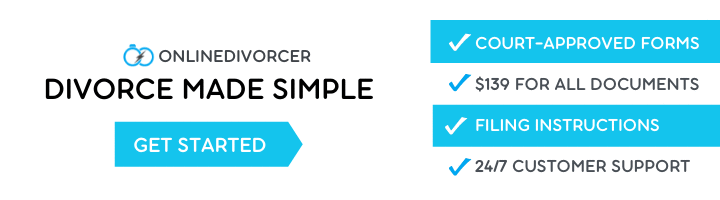
How do I check if a value exists in a list?
To check if the item exists in the list, use Python “in operator”. For example, we can use the “in” operator with the if condition, and if the item exists in the list, then the condition returns True, and if not, then it returns False.
How do you check does not contain in C#?
you can use not operator for check enter value is not in list .
- if (!myList. Contains(“name”))
- {
- myList. Add(“name”);
- }
Why LINQ is used in C#?
LINQ in C# is used to work with data access from sources such as objects, data sets, SQL Server, and XML. LINQ stands for Language Integrated Query. LINQ is a data querying API with SQL like query syntaxes. LINQ provides functions to query cached data from all kinds of data sources.
What is the difference between any and all in LINQ?
All() determines whether all elements of a sequence satisfy a condition. Any() determines whether any element of a sequence satisfies the condition.
Which keyword is use to check if the item is present in the list?
To verify if a specified item is exist in a list use the in keyword.
How do you check whether an element is present in a tuple?
Explanation
- A tuple is defined, and is displayed on the console.
- The value of ‘N’ is initialized.
- The loop is iterated over, and if the element ‘N’ is present in the tuple, a value is assigned ‘True’.
- This value is assigned to a result.
- It is displayed as output on the console.
When should I use IEquatable?
The IEquatable(T) interface is used by generic collection objects such as Dictionary(TKey, TValue) , List(T) , and LinkedList(T) when testing for equality in such methods as Contains , IndexOf , LastIndexOf , and Remove .
What is IEqualityComparer C#?
IEqualityComparer is a very important interface for comparer tasks in the LinQ world. The next extended methods have an overload with this parameter type: Contains, Distinct, Except, Intersect, GrouBy, GroupJoin, Join, SecuenceEqual, ToDictionary, ToLookUp and Union.
How do I check if a value is in a list in Excel?
Select a blank cell, here is C2, and type this formula =IF(ISNUMBER(MATCH(B2,A:A,0)),1,0) into it, and press Enter key to get the result, and if it displays 1, indicates the value is in the list, and if 0, that is not exist.
How do you check to if a string does not contain a string C#?
string. Contains() method returns true if given substring is exists in the string or not else it will return false.
Why LINQ is required?
Readable code: LINQ makes the code more readable so other developers can easily understand and maintain it. Standardized way of querying multiple data sources: The same LINQ syntax can be used to query multiple data sources. Compile time safety of queries: It provides type checking of objects at compile time.