How do you check if an object is of a certain type C#?
Table of Contents
How do you check if an object is of a certain type C#?
To determine whether an object is a specific type, you can use your language’s type comparison keyword or construct. For example, you can use the TypeOf… Is construct in Visual Basic or the is keyword in C#. The GetType method is inherited by all types that derive from Object.
How do I know my dynamic type?
The dynamic type checking is done during program execution. The dynamic type checking is generally executed by saving a type tag in each data object that expresses the data type of the object. For example, an integer data object can include both the integer value and an integer type tag.
How do you find a type object?

Get and print the type of an object: type() type() returns the type of an object. You can use this to get and print the type of a variable and a literal like typeof in other programming languages. The return value of type() is type object such as str or int .
What is the object type in C#?
The object type is an alias for System. Object in . NET. In the unified type system of C#, all types, predefined and user-defined, reference types and value types, inherit directly or indirectly from System.
Which operator is used to check the object has the specified type?
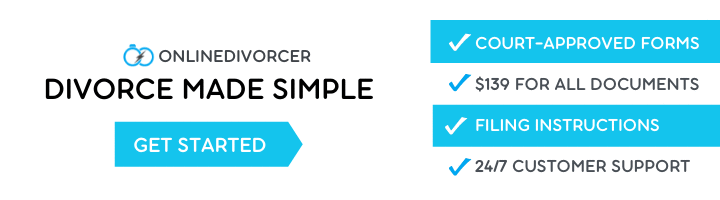
instanceof”
The java “instanceof” operator is used to test whether the object is an instance of the specified type (class or subclass or interface). It is also known as type comparison operator because it compares the instance with type.
What is the type of an object?
An object type is a user-defined composite datatype that encapsulates a data structure along with the functions and procedures needed to manipulate the data. The variables that form the data structure are called attributes.
What method is used to find the data type of a variable?
Type () function is used to know the data type of a variable in python.
What is the difference between typeof and GetType in C#?
typeof keyword takes the Type itself as an argument and returns the underline Type of the argument whereas GetType() can only be invoked on the instance of the type.
What is value type and reference type in C#?
Value types and reference types are the two main categories of C# types. A variable of a value type contains an instance of the type. This differs from a variable of a reference type, which contains a reference to an instance of the type.
How do you check if an object belongs to a certain class?
The java “instanceof” operator is used to test whether the object is an instance of the specified type (class or subclass or interface). It is also known as type comparison operator because it compares the instance with type. It returns either true or false.
What does Instanceof operator do?
The instanceof operator in Java is used to check whether an object is an instance of a particular class or not. objectName instanceOf className; Here, if objectName is an instance of className , the operator returns true . Otherwise, it returns false .
How do you use typeof if statements?
“using typeof in if statement javascript” Code Answer
- // check if a variable is of a certain type.
- var myVar = “This is a string”;
-
- if (typeof myVar === “string”) {
- console. log(“It’s a string!” );
- } else if (typeof myVar === “number”) {
- console. log(“It’s a number!” );
- };
What is typeof for class?
The typeof is an operator keyword which is used to get a type at the compile-time. Or in other words, this operator is used to get the System. Type object for a type. This operator takes the Type itself as an argument and returns the marked type of the argument.