How do you compare two objects in arrays?
Table of Contents
How do you compare two objects in arrays?
To properly compare two arrays or objects, we need to check:
- That they’re the same object type (array vs. object).
- That they have the same number of items.
- That each item is equal to its counterpart in the other array or object. That they’re the same object type (array vs. object vs. string vs. number vs. function).
How do I compare two arrays of objects in es6?
“compare two array of objects javascript es6” Code Answer’s
- var result = result1. filter(function (o1) {
- return result2. some(function (o2) {
- return o1. id === o2. id; // return the ones with equal id.
- });
- });
- // if you want to be more clever…
- let result = result1. filter(o1 => result2. some(o2 => o1. id === o2. id));
How do you compare two elements in an array JavaScript?
Compare out of order array elements In order to compare array elements that are out of order, you can use the combination of every() and includes() method. The includes() method are added into JavaScript on ES7, so if you need to support older JavaScript versions, you can use indexOf() method as an alternative.

Can you compare two arrays in JavaScript?
While JavaScript does not have an inbuilt method to directly compare two arrays, it does have inbuilt methods to compare two strings. Strings can also be compared using the equality operator. Therefore, we can convert the arrays to strings, using the Array join() method, and then check if the strings are equal.
How do you compare two objects?
The equals() method of the Object class compare the equality of two objects. The two objects will be equal if they share the same memory address. Syntax: public boolean equals(Object obj)
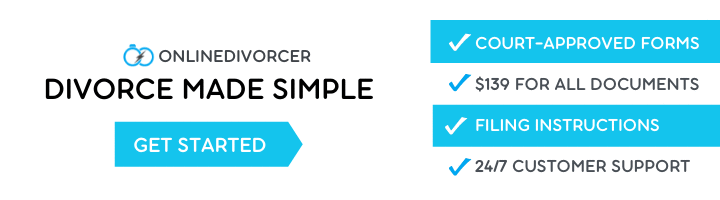
How will you differentiate between arrays and objects in JavaScript?
Both objects and arrays are considered “special” in JavaScript. Objects represent a special data type that is mutable and can be used to store a collection of data (rather than just a single value). Arrays are a special type of variable that is also mutable and can also be used to store a list of values.
How do you compare objects in JavaScript?
Comparing objects is easy, use === or Object.is(). This function returns true if they have the same reference and false if they do not. Again, let me stress, it is comparing the references to the objects, not the keys and values of the objects. So, from Example 3, Object.is(obj1,obj2); would return false.
How can I find matching values in two arrays?
- First of declaring two arrays with elements.
- Declare a function, which is used to compare two arrays and find matches. first of all, Declare an empty array name arr. next split array by commas using split() method.
- Call above defined function with arguments(arr1 and arr2).
- Print result using Console. log().
How do I compare two arrays of arrays?
How to compare two arrays in Java?
- Using Arrays. equals(array1, array2) methods − This method iterates over each value of an array and compare using equals method.
- Using Arrays. deepEquals(array1, array2) methods − This method iterates over each value of an array and deep compare using any overridden equals method.
What is the difference between == and === in JavaScript?
The main difference between the == and === operator in javascript is that the == operator does the type conversion of the operands before comparison, whereas the === operator compares the values as well as the data types of the operands.
Can we compare 2 objects in JavaScript?
Objects are not like arrays or strings. So simply comparing by using “===” or “==” is not possible. Here to compare we have to first stringify the object and then using equality operators it is possible to compare the objects.
How do I compare two array objects in TypeScript?
To get the difference between two arrays of objects:
- Use the filter() method to iterate over the first array.
- Check if each object is not contained in the second array.
- Repeat steps 1 and 2 for the second array.
- Concatenate the results to get the complete difference.
Which function finds out difference between two arrays?
Find difference between two arrays in JavaScript
- Using Array.prototype.filter() function. You can use the filter() method to find the elements of the first array which are not in the second array.
- Using jQuery. With jQuery, you can use the .not() method to get the difference.
- Using Underscore/Lodash Library.
What is the difference between i ++ and I –?
The only difference is the order of operations between the increment of the variable and the value the operator returns. So basically ++i returns the value after it is incremented, while i++ return the value before it is incremented. At the end, in both cases the i will have its value incremented.
What is difference between typeof and Instanceof?
typeof: Per the MDN docmentation, typeof is a unary operator that returns a string indicating the type of the unevaluated operand. instanceof: is a binary operator, accepting an object and a constructor. It returns a boolean indicating whether or not the object has the given constructor in its prototype chain.